Note
Go to the end to download the full example code
Segmentation tutorial
This example demonstrates image segmentation with an Akida-compatible model as illustrated through person segmentation using the Portrait128 dataset.
Using pre-trained models for quick runtime, this example shows the evolution of model performance for a trained keras floating point model, a keras quantized and Quantization Aware Trained (QAT) model, and an Akida-converted model. Notice that the performance of the original keras floating point model is maintained throughout the model conversion flow.
1. Load the dataset
import os
import numpy as np
from akida_models import fetch_file
# Download validation set from Brainchip data server, it contains 10% of the original dataset
data_path = fetch_file(fname="val.tar.gz",
origin="https://data.brainchip.com/dataset-mirror/portrait128/val.tar.gz",
cache_subdir=os.path.join("datasets", "portrait128"),
extract=True)
data_dir = os.path.join(os.path.dirname(data_path), "val")
x_val = np.load(os.path.join(data_dir, "val_img.npy"))
y_val = np.load(os.path.join(data_dir, "val_msk.npy")).astype('uint8')
batch_size = 32
steps = x_val.shape[0] // 32
# Visualize some data
import matplotlib.pyplot as plt
id = np.random.randint(0, x_val.shape[0])
fig, axs = plt.subplots(3, 3, constrained_layout=True)
for col in range(3):
axs[0, col].imshow(x_val[id + col] / 255.)
axs[0, col].axis('off')
axs[1, col].imshow(1 - y_val[id + col], cmap='Greys')
axs[1, col].axis('off')
axs[2, col].imshow(x_val[id + col] / 255. * y_val[id + col])
axs[2, col].axis('off')
fig.suptitle('Image, mask and masked image', fontsize=10)
plt.show()
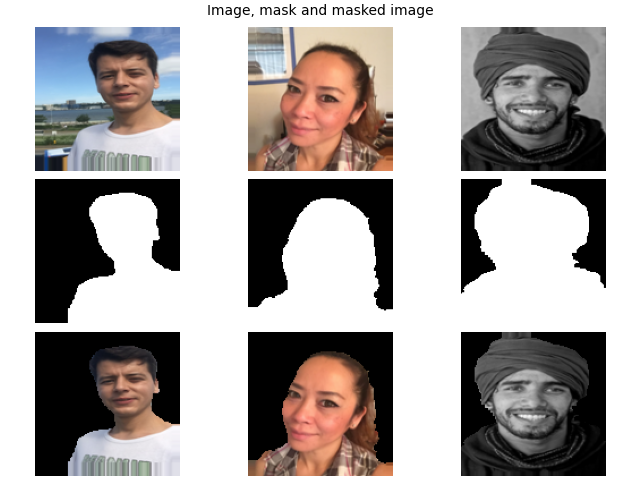
Downloading data from https://data.brainchip.com/dataset-mirror/portrait128/val.tar.gz.
0/267313385 [..............................] - ETA: 0s
122880/267313385 [..............................] - ETA: 1:52
638976/267313385 [..............................] - ETA: 42s
1220608/267313385 [..............................] - ETA: 33s
1802240/267313385 [..............................] - ETA: 29s
2269184/267313385 [..............................] - ETA: 29s
2539520/267313385 [..............................] - ETA: 31s
3104768/267313385 [..............................] - ETA: 30s
3694592/267313385 [..............................] - ETA: 29s
4276224/267313385 [..............................] - ETA: 28s
4882432/267313385 [..............................] - ETA: 27s
5464064/267313385 [..............................] - ETA: 26s
6037504/267313385 [..............................] - ETA: 26s
6627328/267313385 [..............................] - ETA: 25s
7217152/267313385 [..............................] - ETA: 25s
7806976/267313385 [..............................] - ETA: 25s
8396800/267313385 [..............................] - ETA: 25s
9003008/267313385 [>.............................] - ETA: 24s
9592832/267313385 [>.............................] - ETA: 24s
10141696/267313385 [>.............................] - ETA: 24s
10780672/267313385 [>.............................] - ETA: 24s
11386880/267313385 [>.............................] - ETA: 23s
11976704/267313385 [>.............................] - ETA: 23s
12566528/267313385 [>.............................] - ETA: 23s
13156352/267313385 [>.............................] - ETA: 23s
13746176/267313385 [>.............................] - ETA: 23s
14336000/267313385 [>.............................] - ETA: 23s
14925824/267313385 [>.............................] - ETA: 23s
15515648/267313385 [>.............................] - ETA: 23s
16089088/267313385 [>.............................] - ETA: 22s
16678912/267313385 [>.............................] - ETA: 22s
17268736/267313385 [>.............................] - ETA: 22s
17858560/267313385 [=>............................] - ETA: 22s
18448384/267313385 [=>............................] - ETA: 22s
19038208/267313385 [=>............................] - ETA: 22s
19628032/267313385 [=>............................] - ETA: 22s
20217856/267313385 [=>............................] - ETA: 22s
20807680/267313385 [=>............................] - ETA: 22s
21397504/267313385 [=>............................] - ETA: 22s
21987328/267313385 [=>............................] - ETA: 21s
22577152/267313385 [=>............................] - ETA: 21s
23166976/267313385 [=>............................] - ETA: 21s
23756800/267313385 [=>............................] - ETA: 21s
24346624/267313385 [=>............................] - ETA: 21s
24936448/267313385 [=>............................] - ETA: 21s
25526272/267313385 [=>............................] - ETA: 21s
26116096/267313385 [=>............................] - ETA: 21s
26705920/267313385 [=>............................] - ETA: 21s
27295744/267313385 [==>...........................] - ETA: 21s
27885568/267313385 [==>...........................] - ETA: 21s
28475392/267313385 [==>...........................] - ETA: 21s
29065216/267313385 [==>...........................] - ETA: 21s
29655040/267313385 [==>...........................] - ETA: 21s
30228480/267313385 [==>...........................] - ETA: 22s
31096832/267313385 [==>...........................] - ETA: 21s
32079872/267313385 [==>...........................] - ETA: 21s
33865728/267313385 [==>...........................] - ETA: 20s
34455552/267313385 [==>...........................] - ETA: 20s
35045376/267313385 [==>...........................] - ETA: 20s
35635200/267313385 [==>...........................] - ETA: 20s
36225024/267313385 [===>..........................] - ETA: 20s
36814848/267313385 [===>..........................] - ETA: 20s
37404672/267313385 [===>..........................] - ETA: 20s
37994496/267313385 [===>..........................] - ETA: 20s
38584320/267313385 [===>..........................] - ETA: 20s
39174144/267313385 [===>..........................] - ETA: 20s
39763968/267313385 [===>..........................] - ETA: 19s
40353792/267313385 [===>..........................] - ETA: 19s
40943616/267313385 [===>..........................] - ETA: 19s
41533440/267313385 [===>..........................] - ETA: 19s
42090496/267313385 [===>..........................] - ETA: 19s
42647552/267313385 [===>..........................] - ETA: 19s
43204608/267313385 [===>..........................] - ETA: 19s
43761664/267313385 [===>..........................] - ETA: 19s
44318720/267313385 [===>..........................] - ETA: 19s
44859392/267313385 [====>.........................] - ETA: 19s
45416448/267313385 [====>.........................] - ETA: 19s
45957120/267313385 [====>.........................] - ETA: 19s
46530560/267313385 [====>.........................] - ETA: 19s
47087616/267313385 [====>.........................] - ETA: 19s
47644672/267313385 [====>.........................] - ETA: 19s
48201728/267313385 [====>.........................] - ETA: 19s
48791552/267313385 [====>.........................] - ETA: 19s
49381376/267313385 [====>.........................] - ETA: 19s
49905664/267313385 [====>.........................] - ETA: 19s
50085888/267313385 [====>.........................] - ETA: 19s
51200000/267313385 [====>.........................] - ETA: 19s
52559872/267313385 [====>.........................] - ETA: 19s
53018624/267313385 [====>.........................] - ETA: 19s
53608448/267313385 [=====>........................] - ETA: 19s
54198272/267313385 [=====>........................] - ETA: 18s
54788096/267313385 [=====>........................] - ETA: 18s
55361536/267313385 [=====>........................] - ETA: 18s
55951360/267313385 [=====>........................] - ETA: 18s
56541184/267313385 [=====>........................] - ETA: 18s
57131008/267313385 [=====>........................] - ETA: 18s
57720832/267313385 [=====>........................] - ETA: 18s
58310656/267313385 [=====>........................] - ETA: 18s
58900480/267313385 [=====>........................] - ETA: 18s
59490304/267313385 [=====>........................] - ETA: 18s
60080128/267313385 [=====>........................] - ETA: 18s
60669952/267313385 [=====>........................] - ETA: 18s
61243392/267313385 [=====>........................] - ETA: 18s
61833216/267313385 [=====>........................] - ETA: 18s
62423040/267313385 [======>.......................] - ETA: 18s
62947328/267313385 [======>.......................] - ETA: 18s
63291392/267313385 [======>.......................] - ETA: 18s
63881216/267313385 [======>.......................] - ETA: 18s
64454656/267313385 [======>.......................] - ETA: 18s
65044480/267313385 [======>.......................] - ETA: 17s
65634304/267313385 [======>.......................] - ETA: 17s
66224128/267313385 [======>.......................] - ETA: 17s
66584576/267313385 [======>.......................] - ETA: 17s
67141632/267313385 [======>.......................] - ETA: 17s
67731456/267313385 [======>.......................] - ETA: 17s
68321280/267313385 [======>.......................] - ETA: 17s
68911104/267313385 [======>.......................] - ETA: 17s
69500928/267313385 [======>.......................] - ETA: 17s
70041600/267313385 [======>.......................] - ETA: 17s
70582272/267313385 [======>.......................] - ETA: 17s
71122944/267313385 [======>.......................] - ETA: 17s
71663616/267313385 [=======>......................] - ETA: 17s
72187904/267313385 [=======>......................] - ETA: 17s
72777728/267313385 [=======>......................] - ETA: 17s
73367552/267313385 [=======>......................] - ETA: 17s
73957376/267313385 [=======>......................] - ETA: 17s
74547200/267313385 [=======>......................] - ETA: 17s
75120640/267313385 [=======>......................] - ETA: 17s
75661312/267313385 [=======>......................] - ETA: 17s
76201984/267313385 [=======>......................] - ETA: 17s
76742656/267313385 [=======>......................] - ETA: 16s
77266944/267313385 [=======>......................] - ETA: 16s
77791232/267313385 [=======>......................] - ETA: 16s
78381056/267313385 [=======>......................] - ETA: 16s
78970880/267313385 [=======>......................] - ETA: 16s
79560704/267313385 [=======>......................] - ETA: 16s
80150528/267313385 [=======>......................] - ETA: 16s
80740352/267313385 [========>.....................] - ETA: 16s
81281024/267313385 [========>.....................] - ETA: 16s
81821696/267313385 [========>.....................] - ETA: 16s
82362368/267313385 [========>.....................] - ETA: 16s
82886656/267313385 [========>.....................] - ETA: 16s
83410944/267313385 [========>.....................] - ETA: 16s
83951616/267313385 [========>.....................] - ETA: 16s
84541440/267313385 [========>.....................] - ETA: 16s
85131264/267313385 [========>.....................] - ETA: 16s
85721088/267313385 [========>.....................] - ETA: 16s
86310912/267313385 [========>.....................] - ETA: 16s
86900736/267313385 [========>.....................] - ETA: 16s
87441408/267313385 [========>.....................] - ETA: 16s
87982080/267313385 [========>.....................] - ETA: 16s
88522752/267313385 [========>.....................] - ETA: 15s
89047040/267313385 [========>.....................] - ETA: 15s
89571328/267313385 [=========>....................] - ETA: 15s
90095616/267313385 [=========>....................] - ETA: 15s
90685440/267313385 [=========>....................] - ETA: 15s
91258880/267313385 [=========>....................] - ETA: 15s
91848704/267313385 [=========>....................] - ETA: 15s
92438528/267313385 [=========>....................] - ETA: 15s
93028352/267313385 [=========>....................] - ETA: 15s
93585408/267313385 [=========>....................] - ETA: 15s
94126080/267313385 [=========>....................] - ETA: 15s
94666752/267313385 [=========>....................] - ETA: 15s
95191040/267313385 [=========>....................] - ETA: 15s
95715328/267313385 [=========>....................] - ETA: 15s
96239616/267313385 [=========>....................] - ETA: 15s
96813056/267313385 [=========>....................] - ETA: 15s
97353728/267313385 [=========>....................] - ETA: 15s
97910784/267313385 [=========>....................] - ETA: 15s
98467840/267313385 [==========>...................] - ETA: 15s
99024896/267313385 [==========>...................] - ETA: 15s
99532800/267313385 [==========>...................] - ETA: 15s
100024320/267313385 [==========>...................] - ETA: 15s
100515840/267313385 [==========>...................] - ETA: 14s
101023744/267313385 [==========>...................] - ETA: 14s
101548032/267313385 [==========>...................] - ETA: 14s
102055936/267313385 [==========>...................] - ETA: 14s
102629376/267313385 [==========>...................] - ETA: 14s
103219200/267313385 [==========>...................] - ETA: 14s
103809024/267313385 [==========>...................] - ETA: 14s
104398848/267313385 [==========>...................] - ETA: 14s
104988672/267313385 [==========>...................] - ETA: 14s
105529344/267313385 [==========>...................] - ETA: 14s
106070016/267313385 [==========>...................] - ETA: 14s
106610688/267313385 [==========>...................] - ETA: 14s
107134976/267313385 [===========>..................] - ETA: 14s
107659264/267313385 [===========>..................] - ETA: 14s
108199936/267313385 [===========>..................] - ETA: 14s
108789760/267313385 [===========>..................] - ETA: 14s
109379584/267313385 [===========>..................] - ETA: 14s
109969408/267313385 [===========>..................] - ETA: 14s
110559232/267313385 [===========>..................] - ETA: 14s
111149056/267313385 [===========>..................] - ETA: 14s
111689728/267313385 [===========>..................] - ETA: 13s
112230400/267313385 [===========>..................] - ETA: 13s
112754688/267313385 [===========>..................] - ETA: 13s
113278976/267313385 [===========>..................] - ETA: 13s
113786880/267313385 [===========>..................] - ETA: 13s
114327552/267313385 [===========>..................] - ETA: 13s
114917376/267313385 [===========>..................] - ETA: 13s
115507200/267313385 [===========>..................] - ETA: 13s
116097024/267313385 [============>.................] - ETA: 13s
116686848/267313385 [============>.................] - ETA: 13s
117260288/267313385 [============>.................] - ETA: 13s
117800960/267313385 [============>.................] - ETA: 13s
118325248/267313385 [============>.................] - ETA: 13s
118849536/267313385 [============>.................] - ETA: 13s
119357440/267313385 [============>.................] - ETA: 13s
119881728/267313385 [============>.................] - ETA: 13s
120471552/267313385 [============>.................] - ETA: 13s
121061376/267313385 [============>.................] - ETA: 13s
121651200/267313385 [============>.................] - ETA: 13s
122241024/267313385 [============>.................] - ETA: 13s
122830848/267313385 [============>.................] - ETA: 13s
123404288/267313385 [============>.................] - ETA: 12s
123994112/267313385 [============>.................] - ETA: 12s
124567552/267313385 [============>.................] - ETA: 12s
125157376/267313385 [=============>................] - ETA: 12s
125747200/267313385 [=============>................] - ETA: 12s
126337024/267313385 [=============>................] - ETA: 12s
126926848/267313385 [=============>................] - ETA: 12s
127516672/267313385 [=============>................] - ETA: 12s
128106496/267313385 [=============>................] - ETA: 12s
128696320/267313385 [=============>................] - ETA: 12s
129269760/267313385 [=============>................] - ETA: 12s
129826816/267313385 [=============>................] - ETA: 12s
130318336/267313385 [=============>................] - ETA: 12s
130809856/267313385 [=============>................] - ETA: 12s
131284992/267313385 [=============>................] - ETA: 12s
131776512/267313385 [=============>................] - ETA: 12s
132268032/267313385 [=============>................] - ETA: 12s
132726784/267313385 [=============>................] - ETA: 12s
133464064/267313385 [=============>................] - ETA: 12s
134037504/267313385 [==============>...............] - ETA: 12s
134627328/267313385 [==============>...............] - ETA: 11s
135200768/267313385 [==============>...............] - ETA: 11s
135790592/267313385 [==============>...............] - ETA: 11s
136380416/267313385 [==============>...............] - ETA: 11s
136970240/267313385 [==============>...............] - ETA: 11s
137543680/267313385 [==============>...............] - ETA: 11s
138117120/267313385 [==============>...............] - ETA: 11s
138674176/267313385 [==============>...............] - ETA: 11s
139247616/267313385 [==============>...............] - ETA: 11s
139821056/267313385 [==============>...............] - ETA: 11s
140394496/267313385 [==============>...............] - ETA: 11s
140967936/267313385 [==============>...............] - ETA: 11s
141541376/267313385 [==============>...............] - ETA: 11s
142114816/267313385 [==============>...............] - ETA: 11s
142688256/267313385 [===============>..............] - ETA: 11s
143261696/267313385 [===============>..............] - ETA: 11s
143835136/267313385 [===============>..............] - ETA: 11s
144408576/267313385 [===============>..............] - ETA: 11s
144998400/267313385 [===============>..............] - ETA: 11s
145571840/267313385 [===============>..............] - ETA: 10s
146145280/267313385 [===============>..............] - ETA: 10s
146718720/267313385 [===============>..............] - ETA: 10s
147275776/267313385 [===============>..............] - ETA: 10s
147849216/267313385 [===============>..............] - ETA: 10s
148439040/267313385 [===============>..............] - ETA: 10s
149028864/267313385 [===============>..............] - ETA: 10s
149618688/267313385 [===============>..............] - ETA: 10s
150208512/267313385 [===============>..............] - ETA: 10s
150781952/267313385 [===============>..............] - ETA: 10s
151388160/267313385 [===============>..............] - ETA: 10s
151961600/267313385 [================>.............] - ETA: 10s
152518656/267313385 [================>.............] - ETA: 10s
153075712/267313385 [================>.............] - ETA: 10s
153632768/267313385 [================>.............] - ETA: 10s
154189824/267313385 [================>.............] - ETA: 10s
154730496/267313385 [================>.............] - ETA: 10s
155238400/267313385 [================>.............] - ETA: 10s
155893760/267313385 [================>.............] - ETA: 10s
156450816/267313385 [================>.............] - ETA: 9s
157007872/267313385 [================>.............] - ETA: 9s
157564928/267313385 [================>.............] - ETA: 9s
158121984/267313385 [================>.............] - ETA: 9s
158679040/267313385 [================>.............] - ETA: 9s
159268864/267313385 [================>.............] - ETA: 9s
159858688/267313385 [================>.............] - ETA: 9s
160448512/267313385 [=================>............] - ETA: 9s
161038336/267313385 [=================>............] - ETA: 9s
161628160/267313385 [=================>............] - ETA: 9s
162217984/267313385 [=================>............] - ETA: 9s
162807808/267313385 [=================>............] - ETA: 9s
163397632/267313385 [=================>............] - ETA: 9s
163971072/267313385 [=================>............] - ETA: 9s
164511744/267313385 [=================>............] - ETA: 9s
165052416/267313385 [=================>............] - ETA: 9s
165576704/267313385 [=================>............] - ETA: 9s
166100992/267313385 [=================>............] - ETA: 9s
166625280/267313385 [=================>............] - ETA: 9s
167215104/267313385 [=================>............] - ETA: 8s
167804928/267313385 [=================>............] - ETA: 8s
168394752/267313385 [=================>............] - ETA: 8s
168984576/267313385 [=================>............] - ETA: 8s
169574400/267313385 [==================>...........] - ETA: 8s
170164224/267313385 [==================>...........] - ETA: 8s
170754048/267313385 [==================>...........] - ETA: 8s
171343872/267313385 [==================>...........] - ETA: 8s
171933696/267313385 [==================>...........] - ETA: 8s
172523520/267313385 [==================>...........] - ETA: 8s
173113344/267313385 [==================>...........] - ETA: 8s
173703168/267313385 [==================>...........] - ETA: 8s
174292992/267313385 [==================>...........] - ETA: 8s
174882816/267313385 [==================>...........] - ETA: 8s
175472640/267313385 [==================>...........] - ETA: 8s
176029696/267313385 [==================>...........] - ETA: 8s
176619520/267313385 [==================>...........] - ETA: 8s
177209344/267313385 [==================>...........] - ETA: 8s
177799168/267313385 [==================>...........] - ETA: 8s
178388992/267313385 [===================>..........] - ETA: 7s
178978816/267313385 [===================>..........] - ETA: 7s
179568640/267313385 [===================>..........] - ETA: 7s
180158464/267313385 [===================>..........] - ETA: 7s
180748288/267313385 [===================>..........] - ETA: 7s
181338112/267313385 [===================>..........] - ETA: 7s
181927936/267313385 [===================>..........] - ETA: 7s
182517760/267313385 [===================>..........] - ETA: 7s
183107584/267313385 [===================>..........] - ETA: 7s
183697408/267313385 [===================>..........] - ETA: 7s
184287232/267313385 [===================>..........] - ETA: 7s
184860672/267313385 [===================>..........] - ETA: 7s
185466880/267313385 [===================>..........] - ETA: 7s
186056704/267313385 [===================>..........] - ETA: 7s
186646528/267313385 [===================>..........] - ETA: 7s
187236352/267313385 [====================>.........] - ETA: 7s
187826176/267313385 [====================>.........] - ETA: 7s
188416000/267313385 [====================>.........] - ETA: 7s
189005824/267313385 [====================>.........] - ETA: 6s
189595648/267313385 [====================>.........] - ETA: 6s
190185472/267313385 [====================>.........] - ETA: 6s
190775296/267313385 [====================>.........] - ETA: 6s
191365120/267313385 [====================>.........] - ETA: 6s
191954944/267313385 [====================>.........] - ETA: 6s
192544768/267313385 [====================>.........] - ETA: 6s
193134592/267313385 [====================>.........] - ETA: 6s
193724416/267313385 [====================>.........] - ETA: 6s
194314240/267313385 [====================>.........] - ETA: 6s
194904064/267313385 [====================>.........] - ETA: 6s
195493888/267313385 [====================>.........] - ETA: 6s
196083712/267313385 [=====================>........] - ETA: 6s
196673536/267313385 [=====================>........] - ETA: 6s
197263360/267313385 [=====================>........] - ETA: 6s
197853184/267313385 [=====================>........] - ETA: 6s
198443008/267313385 [=====================>........] - ETA: 6s
199032832/267313385 [=====================>........] - ETA: 6s
199573504/267313385 [=====================>........] - ETA: 6s
200097792/267313385 [=====================>........] - ETA: 5s
200638464/267313385 [=====================>........] - ETA: 5s
201162752/267313385 [=====================>........] - ETA: 5s
201687040/267313385 [=====================>........] - ETA: 5s
202244096/267313385 [=====================>........] - ETA: 5s
202833920/267313385 [=====================>........] - ETA: 5s
203423744/267313385 [=====================>........] - ETA: 5s
204013568/267313385 [=====================>........] - ETA: 5s
204603392/267313385 [=====================>........] - ETA: 5s
205193216/267313385 [======================>.......] - ETA: 5s
205783040/267313385 [======================>.......] - ETA: 5s
206372864/267313385 [======================>.......] - ETA: 5s
206962688/267313385 [======================>.......] - ETA: 5s
207552512/267313385 [======================>.......] - ETA: 5s
208142336/267313385 [======================>.......] - ETA: 5s
208732160/267313385 [======================>.......] - ETA: 5s
209321984/267313385 [======================>.......] - ETA: 5s
209911808/267313385 [======================>.......] - ETA: 5s
210501632/267313385 [======================>.......] - ETA: 5s
211091456/267313385 [======================>.......] - ETA: 5s
211681280/267313385 [======================>.......] - ETA: 4s
212271104/267313385 [======================>.......] - ETA: 4s
212860928/267313385 [======================>.......] - ETA: 4s
213450752/267313385 [======================>.......] - ETA: 4s
214040576/267313385 [=======================>......] - ETA: 4s
214630400/267313385 [=======================>......] - ETA: 4s
215220224/267313385 [=======================>......] - ETA: 4s
215810048/267313385 [=======================>......] - ETA: 4s
216399872/267313385 [=======================>......] - ETA: 4s
216989696/267313385 [=======================>......] - ETA: 4s
217579520/267313385 [=======================>......] - ETA: 4s
218169344/267313385 [=======================>......] - ETA: 4s
218759168/267313385 [=======================>......] - ETA: 4s
219348992/267313385 [=======================>......] - ETA: 4s
219938816/267313385 [=======================>......] - ETA: 4s
220528640/267313385 [=======================>......] - ETA: 4s
221118464/267313385 [=======================>......] - ETA: 4s
221708288/267313385 [=======================>......] - ETA: 4s
222298112/267313385 [=======================>......] - ETA: 4s
222887936/267313385 [========================>.....] - ETA: 3s
223477760/267313385 [========================>.....] - ETA: 3s
224067584/267313385 [========================>.....] - ETA: 3s
224673792/267313385 [========================>.....] - ETA: 3s
225263616/267313385 [========================>.....] - ETA: 3s
225853440/267313385 [========================>.....] - ETA: 3s
226443264/267313385 [========================>.....] - ETA: 3s
227033088/267313385 [========================>.....] - ETA: 3s
227622912/267313385 [========================>.....] - ETA: 3s
228212736/267313385 [========================>.....] - ETA: 3s
228802560/267313385 [========================>.....] - ETA: 3s
229392384/267313385 [========================>.....] - ETA: 3s
229982208/267313385 [========================>.....] - ETA: 3s
230572032/267313385 [========================>.....] - ETA: 3s
231161856/267313385 [========================>.....] - ETA: 3s
231768064/267313385 [=========================>....] - ETA: 3s
232357888/267313385 [=========================>....] - ETA: 3s
232947712/267313385 [=========================>....] - ETA: 3s
233537536/267313385 [=========================>....] - ETA: 2s
234127360/267313385 [=========================>....] - ETA: 2s
234684416/267313385 [=========================>....] - ETA: 2s
235208704/267313385 [=========================>....] - ETA: 2s
235454464/267313385 [=========================>....] - ETA: 2s
236388352/267313385 [=========================>....] - ETA: 2s
237748224/267313385 [=========================>....] - ETA: 2s
238714880/267313385 [=========================>....] - ETA: 2s
239304704/267313385 [=========================>....] - ETA: 2s
239894528/267313385 [=========================>....] - ETA: 2s
240467968/267313385 [=========================>....] - ETA: 2s
241057792/267313385 [==========================>...] - ETA: 2s
241647616/267313385 [==========================>...] - ETA: 2s
242237440/267313385 [==========================>...] - ETA: 2s
242827264/267313385 [==========================>...] - ETA: 2s
243417088/267313385 [==========================>...] - ETA: 2s
244006912/267313385 [==========================>...] - ETA: 2s
244596736/267313385 [==========================>...] - ETA: 2s
245137408/267313385 [==========================>...] - ETA: 1s
245727232/267313385 [==========================>...] - ETA: 1s
246317056/267313385 [==========================>...] - ETA: 1s
246906880/267313385 [==========================>...] - ETA: 1s
247496704/267313385 [==========================>...] - ETA: 1s
248086528/267313385 [==========================>...] - ETA: 1s
248676352/267313385 [==========================>...] - ETA: 1s
249233408/267313385 [==========================>...] - ETA: 1s
249823232/267313385 [===========================>..] - ETA: 1s
250413056/267313385 [===========================>..] - ETA: 1s
251002880/267313385 [===========================>..] - ETA: 1s
251592704/267313385 [===========================>..] - ETA: 1s
252182528/267313385 [===========================>..] - ETA: 1s
252772352/267313385 [===========================>..] - ETA: 1s
253362176/267313385 [===========================>..] - ETA: 1s
253952000/267313385 [===========================>..] - ETA: 1s
254541824/267313385 [===========================>..] - ETA: 1s
255131648/267313385 [===========================>..] - ETA: 1s
255705088/267313385 [===========================>..] - ETA: 1s
256278528/267313385 [===========================>..] - ETA: 0s
256868352/267313385 [===========================>..] - ETA: 0s
257458176/267313385 [===========================>..] - ETA: 0s
258048000/267313385 [===========================>..] - ETA: 0s
258637824/267313385 [============================>.] - ETA: 0s
259211264/267313385 [============================>.] - ETA: 0s
259801088/267313385 [============================>.] - ETA: 0s
260390912/267313385 [============================>.] - ETA: 0s
260980736/267313385 [============================>.] - ETA: 0s
261570560/267313385 [============================>.] - ETA: 0s
262160384/267313385 [============================>.] - ETA: 0s
262750208/267313385 [============================>.] - ETA: 0s
263340032/267313385 [============================>.] - ETA: 0s
263929856/267313385 [============================>.] - ETA: 0s
264519680/267313385 [============================>.] - ETA: 0s
265109504/267313385 [============================>.] - ETA: 0s
265699328/267313385 [============================>.] - ETA: 0s
266289152/267313385 [============================>.] - ETA: 0s
266878976/267313385 [============================>.] - ETA: 0s
267313385/267313385 [==============================] - 24s 0us/step
2. Load a pre-trained native Keras model
The model used in this example is AkidaUNet. It has an AkidaNet (0.5) backbone to extract features combined with a succession of separable transposed convolutional blocks to build an image segmentation map. A pre-trained floating point keras model is downloaded to save training time.
Note
The “transposed” convolutional feature is new in Akida 2.0.
The “separable transposed” operation is realized through the combination of a QuantizeML custom DepthwiseConv2DTranspose layer with a standard pointwise convolution.
The performance of the model is evaluated using both pixel accuracy and Binary IoU. The pixel accuracy describes how well the model can predict the segmentation mask pixel by pixel and the Binary IoU takes into account how close the predicted mask is to the ground truth.
from akida_models.model_io import load_model
# Retrieve the model file from Brainchip data server
model_file = fetch_file(fname="akida_unet_portrait128.h5",
origin="https://data.brainchip.com/models/AkidaV2/akida_unet/akida_unet_portrait128.h5",
cache_subdir='models')
# Load the native Keras pre-trained model
model_keras = load_model(model_file)
model_keras.summary()
Downloading data from https://data.brainchip.com/models/AkidaV2/akida_unet/akida_unet_portrait128.h5.
0/4493952 [..............................] - ETA: 0s
196608/4493952 [>.............................] - ETA: 1s
770048/4493952 [====>.........................] - ETA: 0s
1359872/4493952 [========>.....................] - ETA: 0s
1933312/4493952 [===========>..................] - ETA: 0s
2531328/4493952 [===============>..............] - ETA: 0s
3129344/4493952 [===================>..........] - ETA: 0s
3710976/4493952 [=======================>......] - ETA: 0s
4259840/4493952 [===========================>..] - ETA: 0s
4493952/4493952 [==============================] - 0s 0us/step
Model: "akida_unet"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
input (InputLayer) [(None, 128, 128, 3)] 0
rescaling (Rescaling) (None, 128, 128, 3) 0
conv_0 (Conv2D) (None, 64, 64, 16) 432
conv_0/BN (BatchNormalizati (None, 64, 64, 16) 64
on)
conv_0/relu (ReLU) (None, 64, 64, 16) 0
conv_1 (Conv2D) (None, 64, 64, 32) 4608
conv_1/BN (BatchNormalizati (None, 64, 64, 32) 128
on)
conv_1/relu (ReLU) (None, 64, 64, 32) 0
conv_2 (Conv2D) (None, 32, 32, 64) 18432
conv_2/BN (BatchNormalizati (None, 32, 32, 64) 256
on)
conv_2/relu (ReLU) (None, 32, 32, 64) 0
conv_3 (Conv2D) (None, 32, 32, 64) 36864
conv_3/BN (BatchNormalizati (None, 32, 32, 64) 256
on)
conv_3/relu (ReLU) (None, 32, 32, 64) 0
dw_separable_4 (DepthwiseCo (None, 16, 16, 64) 576
nv2D)
pw_separable_4 (Conv2D) (None, 16, 16, 128) 8192
pw_separable_4/BN (BatchNor (None, 16, 16, 128) 512
malization)
pw_separable_4/relu (ReLU) (None, 16, 16, 128) 0
dw_separable_5 (DepthwiseCo (None, 16, 16, 128) 1152
nv2D)
pw_separable_5 (Conv2D) (None, 16, 16, 128) 16384
pw_separable_5/BN (BatchNor (None, 16, 16, 128) 512
malization)
pw_separable_5/relu (ReLU) (None, 16, 16, 128) 0
dw_separable_6 (DepthwiseCo (None, 8, 8, 128) 1152
nv2D)
pw_separable_6 (Conv2D) (None, 8, 8, 256) 32768
pw_separable_6/BN (BatchNor (None, 8, 8, 256) 1024
malization)
pw_separable_6/relu (ReLU) (None, 8, 8, 256) 0
dw_separable_7 (DepthwiseCo (None, 8, 8, 256) 2304
nv2D)
pw_separable_7 (Conv2D) (None, 8, 8, 256) 65536
pw_separable_7/BN (BatchNor (None, 8, 8, 256) 1024
malization)
pw_separable_7/relu (ReLU) (None, 8, 8, 256) 0
dw_separable_8 (DepthwiseCo (None, 8, 8, 256) 2304
nv2D)
pw_separable_8 (Conv2D) (None, 8, 8, 256) 65536
pw_separable_8/BN (BatchNor (None, 8, 8, 256) 1024
malization)
pw_separable_8/relu (ReLU) (None, 8, 8, 256) 0
dw_separable_9 (DepthwiseCo (None, 8, 8, 256) 2304
nv2D)
pw_separable_9 (Conv2D) (None, 8, 8, 256) 65536
pw_separable_9/BN (BatchNor (None, 8, 8, 256) 1024
malization)
pw_separable_9/relu (ReLU) (None, 8, 8, 256) 0
dw_separable_10 (DepthwiseC (None, 8, 8, 256) 2304
onv2D)
pw_separable_10 (Conv2D) (None, 8, 8, 256) 65536
pw_separable_10/BN (BatchNo (None, 8, 8, 256) 1024
rmalization)
pw_separable_10/relu (ReLU) (None, 8, 8, 256) 0
dw_separable_11 (DepthwiseC (None, 8, 8, 256) 2304
onv2D)
pw_separable_11 (Conv2D) (None, 8, 8, 256) 65536
pw_separable_11/BN (BatchNo (None, 8, 8, 256) 1024
rmalization)
pw_separable_11/relu (ReLU) (None, 8, 8, 256) 0
dw_separable_12 (DepthwiseC (None, 4, 4, 256) 2304
onv2D)
pw_separable_12 (Conv2D) (None, 4, 4, 512) 131072
pw_separable_12/BN (BatchNo (None, 4, 4, 512) 2048
rmalization)
pw_separable_12/relu (ReLU) (None, 4, 4, 512) 0
dw_separable_13 (DepthwiseC (None, 4, 4, 512) 4608
onv2D)
pw_separable_13 (Conv2D) (None, 4, 4, 512) 262144
pw_separable_13/BN (BatchNo (None, 4, 4, 512) 2048
rmalization)
pw_separable_13/relu (ReLU) (None, 4, 4, 512) 0
dw_sepconv_t_0 (DepthwiseCo (None, 8, 8, 512) 5120
nv2DTranspose)
pw_sepconv_t_0 (Conv2D) (None, 8, 8, 256) 131328
pw_sepconv_t_0/BN (BatchNor (None, 8, 8, 256) 1024
malization)
pw_sepconv_t_0/relu (ReLU) (None, 8, 8, 256) 0
dropout (Dropout) (None, 8, 8, 256) 0
dw_sepconv_t_1 (DepthwiseCo (None, 16, 16, 256) 2560
nv2DTranspose)
pw_sepconv_t_1 (Conv2D) (None, 16, 16, 128) 32896
pw_sepconv_t_1/BN (BatchNor (None, 16, 16, 128) 512
malization)
pw_sepconv_t_1/relu (ReLU) (None, 16, 16, 128) 0
dropout_1 (Dropout) (None, 16, 16, 128) 0
dw_sepconv_t_2 (DepthwiseCo (None, 32, 32, 128) 1280
nv2DTranspose)
pw_sepconv_t_2 (Conv2D) (None, 32, 32, 64) 8256
pw_sepconv_t_2/BN (BatchNor (None, 32, 32, 64) 256
malization)
pw_sepconv_t_2/relu (ReLU) (None, 32, 32, 64) 0
dropout_2 (Dropout) (None, 32, 32, 64) 0
dw_sepconv_t_3 (DepthwiseCo (None, 64, 64, 64) 640
nv2DTranspose)
pw_sepconv_t_3 (Conv2D) (None, 64, 64, 32) 2080
pw_sepconv_t_3/BN (BatchNor (None, 64, 64, 32) 128
malization)
pw_sepconv_t_3/relu (ReLU) (None, 64, 64, 32) 0
dropout_3 (Dropout) (None, 64, 64, 32) 0
dw_sepconv_t_4 (DepthwiseCo (None, 128, 128, 32) 320
nv2DTranspose)
pw_sepconv_t_4 (Conv2D) (None, 128, 128, 16) 528
pw_sepconv_t_4/BN (BatchNor (None, 128, 128, 16) 64
malization)
pw_sepconv_t_4/relu (ReLU) (None, 128, 128, 16) 0
dropout_4 (Dropout) (None, 128, 128, 16) 0
head (Conv2D) (None, 128, 128, 1) 17
sigmoid_act (Activation) (None, 128, 128, 1) 0
=================================================================
Total params: 1,058,865
Trainable params: 1,051,889
Non-trainable params: 6,976
_________________________________________________________________
from keras.metrics import BinaryIoU
# Compile the native Keras model (required to evaluate the metrics)
model_keras.compile(loss='binary_crossentropy', metrics=[BinaryIoU(), 'accuracy'])
# Check Keras model performance
_, biou, acc = model_keras.evaluate(x_val, y_val, steps=steps, verbose=0)
print(f"Keras binary IoU / pixel accuracy: {biou:.4f} / {100*acc:.2f}%")
Keras binary IoU / pixel accuracy: 0.9455 / 97.28%
3. Load a pre-trained quantized Keras model
The next step is to quantize and potentially perform Quantize Aware Training (QAT) on the Keras model from the previous step. After the Keras model is quantized to 8-bits for all weights and activations, QAT is used to maintain the performance of the quantized model. Again, a pre-trained model is downloaded to save runtime.
from akida_models import akida_unet_portrait128_pretrained
# Load the pre-trained quantized model
model_quantized_keras = akida_unet_portrait128_pretrained()
model_quantized_keras.summary()
Downloading data from https://data.brainchip.com/models/AkidaV2/akida_unet/akida_unet_portrait128_i8_w8_a8.h5.
0/4520576 [..............................] - ETA: 0s
98304/4520576 [..............................] - ETA: 2s
524288/4520576 [==>...........................] - ETA: 0s
1081344/4520576 [======>.......................] - ETA: 0s
1622016/4520576 [=========>....................] - ETA: 0s
2170880/4520576 [=============>................] - ETA: 0s
2727936/4520576 [=================>............] - ETA: 0s
3284992/4520576 [====================>.........] - ETA: 0s
3833856/4520576 [========================>.....] - ETA: 0s
4374528/4520576 [============================>.] - ETA: 0s
4520576/4520576 [==============================] - 0s 0us/step
Model: "akida_unet"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
input (InputLayer) [(None, 128, 128, 3)] 0
rescaling (QuantizedRescali (None, 128, 128, 3) 0
ng)
conv_0 (QuantizedConv2D) (None, 64, 64, 16) 448
conv_0/relu (QuantizedReLU) (None, 64, 64, 16) 32
conv_1 (QuantizedConv2D) (None, 64, 64, 32) 4640
conv_1/relu (QuantizedReLU) (None, 64, 64, 32) 64
conv_2 (QuantizedConv2D) (None, 32, 32, 64) 18496
conv_2/relu (QuantizedReLU) (None, 32, 32, 64) 128
conv_3 (QuantizedConv2D) (None, 32, 32, 64) 36928
conv_3/relu (QuantizedReLU) (None, 32, 32, 64) 128
dw_separable_4 (QuantizedDe (None, 16, 16, 64) 704
pthwiseConv2D)
pw_separable_4 (QuantizedCo (None, 16, 16, 128) 8320
nv2D)
pw_separable_4/relu (Quanti (None, 16, 16, 128) 256
zedReLU)
dw_separable_5 (QuantizedDe (None, 16, 16, 128) 1408
pthwiseConv2D)
pw_separable_5 (QuantizedCo (None, 16, 16, 128) 16512
nv2D)
pw_separable_5/relu (Quanti (None, 16, 16, 128) 256
zedReLU)
dw_separable_6 (QuantizedDe (None, 8, 8, 128) 1408
pthwiseConv2D)
pw_separable_6 (QuantizedCo (None, 8, 8, 256) 33024
nv2D)
pw_separable_6/relu (Quanti (None, 8, 8, 256) 512
zedReLU)
dw_separable_7 (QuantizedDe (None, 8, 8, 256) 2816
pthwiseConv2D)
pw_separable_7 (QuantizedCo (None, 8, 8, 256) 65792
nv2D)
pw_separable_7/relu (Quanti (None, 8, 8, 256) 512
zedReLU)
dw_separable_8 (QuantizedDe (None, 8, 8, 256) 2816
pthwiseConv2D)
pw_separable_8 (QuantizedCo (None, 8, 8, 256) 65792
nv2D)
pw_separable_8/relu (Quanti (None, 8, 8, 256) 512
zedReLU)
dw_separable_9 (QuantizedDe (None, 8, 8, 256) 2816
pthwiseConv2D)
pw_separable_9 (QuantizedCo (None, 8, 8, 256) 65792
nv2D)
pw_separable_9/relu (Quanti (None, 8, 8, 256) 512
zedReLU)
dw_separable_10 (QuantizedD (None, 8, 8, 256) 2816
epthwiseConv2D)
pw_separable_10 (QuantizedC (None, 8, 8, 256) 65792
onv2D)
pw_separable_10/relu (Quant (None, 8, 8, 256) 512
izedReLU)
dw_separable_11 (QuantizedD (None, 8, 8, 256) 2816
epthwiseConv2D)
pw_separable_11 (QuantizedC (None, 8, 8, 256) 65792
onv2D)
pw_separable_11/relu (Quant (None, 8, 8, 256) 512
izedReLU)
dw_separable_12 (QuantizedD (None, 4, 4, 256) 2816
epthwiseConv2D)
pw_separable_12 (QuantizedC (None, 4, 4, 512) 131584
onv2D)
pw_separable_12/relu (Quant (None, 4, 4, 512) 1024
izedReLU)
dw_separable_13 (QuantizedD (None, 4, 4, 512) 5632
epthwiseConv2D)
pw_separable_13 (QuantizedC (None, 4, 4, 512) 262656
onv2D)
pw_separable_13/relu (Quant (None, 4, 4, 512) 1024
izedReLU)
dw_sepconv_t_0 (QuantizedDe (None, 8, 8, 512) 6144
pthwiseConv2DTranspose)
pw_sepconv_t_0 (QuantizedCo (None, 8, 8, 256) 131328
nv2D)
pw_sepconv_t_0/relu (Quanti (None, 8, 8, 256) 512
zedReLU)
dropout (QuantizedDropout) (None, 8, 8, 256) 0
dw_sepconv_t_1 (QuantizedDe (None, 16, 16, 256) 3072
pthwiseConv2DTranspose)
pw_sepconv_t_1 (QuantizedCo (None, 16, 16, 128) 32896
nv2D)
pw_sepconv_t_1/relu (Quanti (None, 16, 16, 128) 256
zedReLU)
dropout_1 (QuantizedDropout (None, 16, 16, 128) 0
)
dw_sepconv_t_2 (QuantizedDe (None, 32, 32, 128) 1536
pthwiseConv2DTranspose)
pw_sepconv_t_2 (QuantizedCo (None, 32, 32, 64) 8256
nv2D)
pw_sepconv_t_2/relu (Quanti (None, 32, 32, 64) 128
zedReLU)
dropout_2 (QuantizedDropout (None, 32, 32, 64) 0
)
dw_sepconv_t_3 (QuantizedDe (None, 64, 64, 64) 768
pthwiseConv2DTranspose)
pw_sepconv_t_3 (QuantizedCo (None, 64, 64, 32) 2080
nv2D)
pw_sepconv_t_3/relu (Quanti (None, 64, 64, 32) 64
zedReLU)
dropout_3 (QuantizedDropout (None, 64, 64, 32) 0
)
dw_sepconv_t_4 (QuantizedDe (None, 128, 128, 32) 384
pthwiseConv2DTranspose)
pw_sepconv_t_4 (QuantizedCo (None, 128, 128, 16) 528
nv2D)
pw_sepconv_t_4/relu (Quanti (None, 128, 128, 16) 32
zedReLU)
dropout_4 (QuantizedDropout (None, 128, 128, 16) 0
)
head (QuantizedConv2D) (None, 128, 128, 1) 17
dequantizer (Dequantizer) (None, 128, 128, 1) 0
sigmoid_act (Activation) (None, 128, 128, 1) 0
=================================================================
Total params: 1,061,601
Trainable params: 1,047,905
Non-trainable params: 13,696
_________________________________________________________________
# Compile the quantized Keras model (required to evaluate the metrics)
model_quantized_keras.compile(loss='binary_crossentropy', metrics=[BinaryIoU(), 'accuracy'])
# Check Keras model performance
_, biou, acc = model_quantized_keras.evaluate(x_val, y_val, steps=steps, verbose=0)
print(f"Keras quantized binary IoU / pixel accuracy: {biou:.4f} / {100*acc:.2f}%")
Keras quantized binary IoU / pixel accuracy: 0.9399 / 97.01%
4. Conversion to Akida
Finally, the quantized Keras model from the previous step is converted into an Akida model and its performance is evaluated. Note that the original performance of the keras floating point model is maintained throughout the conversion process in this example.
from cnn2snn import convert
# Convert the model
model_akida = convert(model_quantized_keras)
model_akida.summary()
Model Summary
_________________________________________________
Input shape Output shape Sequences Layers
=================================================
[128, 128, 3] [128, 128, 1] 1 36
_________________________________________________
_____________________________________________________________________________
Layer (type) Output shape Kernel shape
====================== SW/conv_0-dequantizer (Software) =====================
conv_0 (InputConv2D) [64, 64, 16] (3, 3, 3, 16)
_____________________________________________________________________________
conv_1 (Conv2D) [64, 64, 32] (3, 3, 16, 32)
_____________________________________________________________________________
conv_2 (Conv2D) [32, 32, 64] (3, 3, 32, 64)
_____________________________________________________________________________
conv_3 (Conv2D) [32, 32, 64] (3, 3, 64, 64)
_____________________________________________________________________________
dw_separable_4 (DepthwiseConv2D) [16, 16, 64] (3, 3, 64, 1)
_____________________________________________________________________________
pw_separable_4 (Conv2D) [16, 16, 128] (1, 1, 64, 128)
_____________________________________________________________________________
dw_separable_5 (DepthwiseConv2D) [16, 16, 128] (3, 3, 128, 1)
_____________________________________________________________________________
pw_separable_5 (Conv2D) [16, 16, 128] (1, 1, 128, 128)
_____________________________________________________________________________
dw_separable_6 (DepthwiseConv2D) [8, 8, 128] (3, 3, 128, 1)
_____________________________________________________________________________
pw_separable_6 (Conv2D) [8, 8, 256] (1, 1, 128, 256)
_____________________________________________________________________________
dw_separable_7 (DepthwiseConv2D) [8, 8, 256] (3, 3, 256, 1)
_____________________________________________________________________________
pw_separable_7 (Conv2D) [8, 8, 256] (1, 1, 256, 256)
_____________________________________________________________________________
dw_separable_8 (DepthwiseConv2D) [8, 8, 256] (3, 3, 256, 1)
_____________________________________________________________________________
pw_separable_8 (Conv2D) [8, 8, 256] (1, 1, 256, 256)
_____________________________________________________________________________
dw_separable_9 (DepthwiseConv2D) [8, 8, 256] (3, 3, 256, 1)
_____________________________________________________________________________
pw_separable_9 (Conv2D) [8, 8, 256] (1, 1, 256, 256)
_____________________________________________________________________________
dw_separable_10 (DepthwiseConv2D) [8, 8, 256] (3, 3, 256, 1)
_____________________________________________________________________________
pw_separable_10 (Conv2D) [8, 8, 256] (1, 1, 256, 256)
_____________________________________________________________________________
dw_separable_11 (DepthwiseConv2D) [8, 8, 256] (3, 3, 256, 1)
_____________________________________________________________________________
pw_separable_11 (Conv2D) [8, 8, 256] (1, 1, 256, 256)
_____________________________________________________________________________
dw_separable_12 (DepthwiseConv2D) [4, 4, 256] (3, 3, 256, 1)
_____________________________________________________________________________
pw_separable_12 (Conv2D) [4, 4, 512] (1, 1, 256, 512)
_____________________________________________________________________________
dw_separable_13 (DepthwiseConv2D) [4, 4, 512] (3, 3, 512, 1)
_____________________________________________________________________________
pw_separable_13 (Conv2D) [4, 4, 512] (1, 1, 512, 512)
_____________________________________________________________________________
dw_sepconv_t_0 (DepthwiseConv2DTranspose) [8, 8, 512] (3, 3, 512, 1)
_____________________________________________________________________________
pw_sepconv_t_0 (Conv2D) [8, 8, 256] (1, 1, 512, 256)
_____________________________________________________________________________
dw_sepconv_t_1 (DepthwiseConv2DTranspose) [16, 16, 256] (3, 3, 256, 1)
_____________________________________________________________________________
pw_sepconv_t_1 (Conv2D) [16, 16, 128] (1, 1, 256, 128)
_____________________________________________________________________________
dw_sepconv_t_2 (DepthwiseConv2DTranspose) [32, 32, 128] (3, 3, 128, 1)
_____________________________________________________________________________
pw_sepconv_t_2 (Conv2D) [32, 32, 64] (1, 1, 128, 64)
_____________________________________________________________________________
dw_sepconv_t_3 (DepthwiseConv2DTranspose) [64, 64, 64] (3, 3, 64, 1)
_____________________________________________________________________________
pw_sepconv_t_3 (Conv2D) [64, 64, 32] (1, 1, 64, 32)
_____________________________________________________________________________
dw_sepconv_t_4 (DepthwiseConv2DTranspose) [128, 128, 32] (3, 3, 32, 1)
_____________________________________________________________________________
pw_sepconv_t_4 (Conv2D) [128, 128, 16] (1, 1, 32, 16)
_____________________________________________________________________________
head (Conv2D) [128, 128, 1] (1, 1, 16, 1)
_____________________________________________________________________________
dequantizer (Dequantizer) [128, 128, 1] N/A
_____________________________________________________________________________
import tensorflow as tf
# Check Akida model performance
labels, pots = None, None
for s in range(steps):
batch = x_val[s * batch_size: (s + 1) * batch_size, :]
label_batch = y_val[s * batch_size: (s + 1) * batch_size, :]
pots_batch = model_akida.predict(batch.astype('uint8'))
if labels is None:
labels = label_batch
pots = pots_batch
else:
labels = np.concatenate((labels, label_batch))
pots = np.concatenate((pots, pots_batch))
preds = tf.keras.activations.sigmoid(pots)
m_binary_iou = tf.keras.metrics.BinaryIoU(target_class_ids=[0, 1], threshold=0.5)
m_binary_iou.update_state(labels, preds)
binary_iou = m_binary_iou.result().numpy()
m_accuracy = tf.keras.metrics.Accuracy()
m_accuracy.update_state(labels, preds > 0.5)
accuracy = m_accuracy.result().numpy()
print(f"Akida binary IoU / pixel accuracy: {binary_iou:.4f} / {100*accuracy:.2f}%")
# For non-regression purpose
assert binary_iou > 0.9
Akida binary IoU / pixel accuracy: 0.9388 / 97.01%
5. Segment a single image
For visualization of the person segmentation performed by the Akida model, display a single image along with the segmentation produced by the original floating point model and the ground truth segmentation.
import matplotlib.pyplot as plt
# Estimate age on a random single image and display Keras and Akida outputs
sample = np.expand_dims(x_val[id, :], 0)
keras_out = model_keras(sample)
akida_out = tf.keras.activations.sigmoid(model_akida.forward(sample.astype('uint8')))
fig, axs = plt.subplots(1, 3, constrained_layout=True)
axs[0].imshow(keras_out[0] * sample[0] / 255.)
axs[0].set_title('Keras segmentation', fontsize=10)
axs[0].axis('off')
axs[1].imshow(akida_out[0] * sample[0] / 255.)
axs[1].set_title('Akida segmentation', fontsize=10)
axs[1].axis('off')
axs[2].imshow(y_val[id] * sample[0] / 255.)
axs[2].set_title('Expected segmentation', fontsize=10)
axs[2].axis('off')
plt.show()
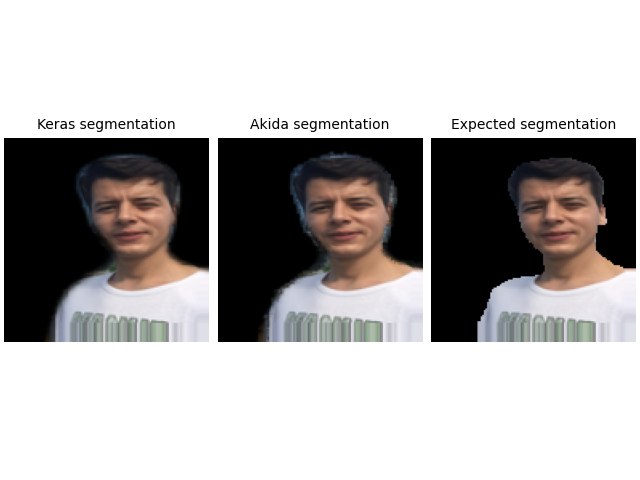
Total running time of the script: (1 minutes 42.545 seconds)