Note
Go to the end to download the full example code
YOLO/PASCAL-VOC detection tutorial
This tutorial demonstrates that Akida can perform object detection. This is illustrated using a subset of the PASCAL-VOC 2007 dataset with “car” and “person” classes only. The YOLOv2 architecture from Redmon et al (2016) has been chosen to tackle this object detection problem.
1. Introduction
1.1 Object detection
Object detection is a computer vision task that combines two elemental tasks:
object classification that consists in assigning a class label to an image like shown in the AkidaNet/ImageNet inference example
object localization that consists in drawing a bounding box around one or several objects in an image
One can learn more about the subject reading this introduction to object detection blog article.
1.2 YOLO key concepts
You Only Look Once (YOLO) is a deep neural network architecture dedicated to object detection.
As opposed to classic networks that handle object detection, YOLO predicts bounding boxes (localization task) and class probabilities (classification task) from a single neural network in a single evaluation. The object detection task is reduced to a regression problem to spatially separated boxes and associated class probabilities.
YOLO base concept is to divide an input image into regions, forming a grid, and to predict bounding boxes and probabilities for each region. The bounding boxes are weighted by the prediction probabilities.
YOLO also uses the concept of “anchors boxes” or “prior boxes”. The network does not actually predict the actual bounding boxes but offsets from anchors boxes which are templates (width/height ratio) computed by clustering the dimensions of the ground truth boxes from the training dataset. The anchors then represent the average shape and size of the objects to detect. More details on the anchors boxes concept are given in this blog article.
Additional information about YOLO can be found on the Darknet website and source code for the preprocessing and postprocessing functions that are included in akida_models package (see the processing section in the model zoo) is largely inspired from experiencor github.
2. Preprocessing tools
As this example focuses on car and person detection only, a subset of VOC has been prepared with test images from VOC2007 that contains at least one of the occurence of the two classes. Just like the VOC dataset, the subset contains an image folder, an annotation folder and a text file listing the file names of interest.
The YOLO toolkit offers several methods to prepare data for processing, see load_image, preprocess_image or parse_voc_annotations.
import os
from tensorflow.keras.utils import get_file
from akida_models.detection.processing import parse_voc_annotations
# Download validation set from Brainchip data server
data_path = get_file(
"voc_test_car_person.tar.gz",
"https://data.brainchip.com/dataset-mirror/voc/voc_test_car_person.tar.gz",
cache_subdir='datasets/voc',
extract=True)
data_dir = os.path.dirname(data_path)
gt_folder = os.path.join(data_dir, 'voc_test_car_person', 'Annotations')
image_folder = os.path.join(data_dir, 'voc_test_car_person', 'JPEGImages')
file_path = os.path.join(
data_dir, 'voc_test_car_person', 'test_car_person.txt')
labels = ['car', 'person']
val_data = parse_voc_annotations(gt_folder, image_folder, file_path, labels)
print("Loaded VOC2007 test data for car and person classes: "
f"{len(val_data)} images.")
Downloading data from https://data.brainchip.com/dataset-mirror/voc/voc_test_car_person.tar.gz
8192/221551911 [..............................] - ETA: 0s
172032/221551911 [..............................] - ETA: 1:04
385024/221551911 [..............................] - ETA: 57s
925696/221551911 [..............................] - ETA: 36s
1318912/221551911 [..............................] - ETA: 33s
1687552/221551911 [..............................] - ETA: 32s
2056192/221551911 [..............................] - ETA: 32s
2441216/221551911 [..............................] - ETA: 31s
2826240/221551911 [..............................] - ETA: 31s
3219456/221551911 [..............................] - ETA: 30s
3612672/221551911 [..............................] - ETA: 30s
4022272/221551911 [..............................] - ETA: 30s
4407296/221551911 [..............................] - ETA: 29s
4833280/221551911 [..............................] - ETA: 29s
5226496/221551911 [..............................] - ETA: 29s
5660672/221551911 [..............................] - ETA: 28s
6086656/221551911 [..............................] - ETA: 28s
6512640/221551911 [..............................] - ETA: 28s
6938624/221551911 [..............................] - ETA: 28s
7348224/221551911 [..............................] - ETA: 28s
7790592/221551911 [>.............................] - ETA: 27s
8232960/221551911 [>.............................] - ETA: 27s
8691712/221551911 [>.............................] - ETA: 27s
9150464/221551911 [>.............................] - ETA: 27s
9609216/221551911 [>.............................] - ETA: 26s
10084352/221551911 [>.............................] - ETA: 26s
10543104/221551911 [>.............................] - ETA: 26s
11034624/221551911 [>.............................] - ETA: 26s
11493376/221551911 [>.............................] - ETA: 25s
11984896/221551911 [>.............................] - ETA: 25s
12476416/221551911 [>.............................] - ETA: 25s
12951552/221551911 [>.............................] - ETA: 25s
13459456/221551911 [>.............................] - ETA: 25s
13950976/221551911 [>.............................] - ETA: 24s
14442496/221551911 [>.............................] - ETA: 24s
14950400/221551911 [=>............................] - ETA: 24s
15458304/221551911 [=>............................] - ETA: 24s
15982592/221551911 [=>............................] - ETA: 24s
16506880/221551911 [=>............................] - ETA: 23s
17031168/221551911 [=>............................] - ETA: 23s
17555456/221551911 [=>............................] - ETA: 23s
18079744/221551911 [=>............................] - ETA: 23s
18587648/221551911 [=>............................] - ETA: 23s
19128320/221551911 [=>............................] - ETA: 23s
19668992/221551911 [=>............................] - ETA: 22s
20209664/221551911 [=>............................] - ETA: 22s
20750336/221551911 [=>............................] - ETA: 22s
21291008/221551911 [=>............................] - ETA: 22s
21848064/221551911 [=>............................] - ETA: 22s
22405120/221551911 [==>...........................] - ETA: 22s
22962176/221551911 [==>...........................] - ETA: 21s
23519232/221551911 [==>...........................] - ETA: 21s
24092672/221551911 [==>...........................] - ETA: 21s
24649728/221551911 [==>...........................] - ETA: 21s
25206784/221551911 [==>...........................] - ETA: 21s
25780224/221551911 [==>...........................] - ETA: 21s
26337280/221551911 [==>...........................] - ETA: 21s
26894336/221551911 [==>...........................] - ETA: 20s
27451392/221551911 [==>...........................] - ETA: 20s
28008448/221551911 [==>...........................] - ETA: 20s
28598272/221551911 [==>...........................] - ETA: 20s
29155328/221551911 [==>...........................] - ETA: 20s
29728768/221551911 [===>..........................] - ETA: 20s
30285824/221551911 [===>..........................] - ETA: 20s
30842880/221551911 [===>..........................] - ETA: 20s
31399936/221551911 [===>..........................] - ETA: 19s
31956992/221551911 [===>..........................] - ETA: 19s
32497664/221551911 [===>..........................] - ETA: 19s
33062912/221551911 [===>..........................] - ETA: 19s
33595392/221551911 [===>..........................] - ETA: 19s
34136064/221551911 [===>..........................] - ETA: 19s
34594816/221551911 [===>..........................] - ETA: 19s
35069952/221551911 [===>..........................] - ETA: 19s
35545088/221551911 [===>..........................] - ETA: 19s
36036608/221551911 [===>..........................] - ETA: 19s
36528128/221551911 [===>..........................] - ETA: 19s
37036032/221551911 [====>.........................] - ETA: 19s
37527552/221551911 [====>.........................] - ETA: 19s
38019072/221551911 [====>.........................] - ETA: 19s
38526976/221551911 [====>.........................] - ETA: 19s
39034880/221551911 [====>.........................] - ETA: 18s
39542784/221551911 [====>.........................] - ETA: 18s
40050688/221551911 [====>.........................] - ETA: 18s
40591360/221551911 [====>.........................] - ETA: 18s
41107456/221551911 [====>.........................] - ETA: 18s
41623552/221551911 [====>.........................] - ETA: 18s
42164224/221551911 [====>.........................] - ETA: 18s
42688512/221551911 [====>.........................] - ETA: 18s
43229184/221551911 [====>.........................] - ETA: 18s
43769856/221551911 [====>.........................] - ETA: 18s
44310528/221551911 [=====>........................] - ETA: 18s
44834816/221551911 [=====>........................] - ETA: 18s
45383680/221551911 [=====>........................] - ETA: 18s
45932544/221551911 [=====>........................] - ETA: 18s
46489600/221551911 [=====>........................] - ETA: 17s
47046656/221551911 [=====>........................] - ETA: 17s
47603712/221551911 [=====>........................] - ETA: 17s
48144384/221551911 [=====>........................] - ETA: 17s
48717824/221551911 [=====>........................] - ETA: 17s
49274880/221551911 [=====>........................] - ETA: 17s
49831936/221551911 [=====>........................] - ETA: 17s
50388992/221551911 [=====>........................] - ETA: 17s
50962432/221551911 [=====>........................] - ETA: 17s
51470336/221551911 [=====>........................] - ETA: 17s
51896320/221551911 [======>.......................] - ETA: 17s
52338688/221551911 [======>.......................] - ETA: 17s
52781056/221551911 [======>.......................] - ETA: 17s
53223424/221551911 [======>.......................] - ETA: 17s
53665792/221551911 [======>.......................] - ETA: 17s
54108160/221551911 [======>.......................] - ETA: 17s
54566912/221551911 [======>.......................] - ETA: 17s
55025664/221551911 [======>.......................] - ETA: 17s
55476224/221551911 [======>.......................] - ETA: 17s
55943168/221551911 [======>.......................] - ETA: 16s
56401920/221551911 [======>.......................] - ETA: 16s
56893440/221551911 [======>.......................] - ETA: 16s
57368576/221551911 [======>.......................] - ETA: 16s
57843712/221551911 [======>.......................] - ETA: 16s
58351616/221551911 [======>.......................] - ETA: 16s
58826752/221551911 [======>.......................] - ETA: 16s
59334656/221551911 [=======>......................] - ETA: 16s
59826176/221551911 [=======>......................] - ETA: 16s
60334080/221551911 [=======>......................] - ETA: 16s
60841984/221551911 [=======>......................] - ETA: 16s
61349888/221551911 [=======>......................] - ETA: 16s
61857792/221551911 [=======>......................] - ETA: 16s
62382080/221551911 [=======>......................] - ETA: 16s
62889984/221551911 [=======>......................] - ETA: 16s
63430656/221551911 [=======>......................] - ETA: 16s
63938560/221551911 [=======>......................] - ETA: 16s
64462848/221551911 [=======>......................] - ETA: 16s
64987136/221551911 [=======>......................] - ETA: 16s
65527808/221551911 [=======>......................] - ETA: 15s
66052096/221551911 [=======>......................] - ETA: 15s
66592768/221551911 [========>.....................] - ETA: 15s
67100672/221551911 [========>.....................] - ETA: 15s
67624960/221551911 [========>.....................] - ETA: 15s
68132864/221551911 [========>.....................] - ETA: 15s
68657152/221551911 [========>.....................] - ETA: 15s
69165056/221551911 [========>.....................] - ETA: 15s
69705728/221551911 [========>.....................] - ETA: 15s
70230016/221551911 [========>.....................] - ETA: 15s
70770688/221551911 [========>.....................] - ETA: 15s
71294976/221551911 [========>.....................] - ETA: 15s
71835648/221551911 [========>.....................] - ETA: 15s
72376320/221551911 [========>.....................] - ETA: 15s
72900608/221551911 [========>.....................] - ETA: 15s
73441280/221551911 [========>.....................] - ETA: 15s
73949184/221551911 [=========>....................] - ETA: 15s
74440704/221551911 [=========>....................] - ETA: 14s
74866688/221551911 [=========>....................] - ETA: 14s
75276288/221551911 [=========>....................] - ETA: 14s
75718656/221551911 [=========>....................] - ETA: 14s
76193792/221551911 [=========>....................] - ETA: 14s
76636160/221551911 [=========>....................] - ETA: 14s
77078528/221551911 [=========>....................] - ETA: 14s
77570048/221551911 [=========>....................] - ETA: 14s
78028800/221551911 [=========>....................] - ETA: 14s
78512128/221551911 [=========>....................] - ETA: 14s
78995456/221551911 [=========>....................] - ETA: 14s
79486976/221551911 [=========>....................] - ETA: 14s
79962112/221551911 [=========>....................] - ETA: 14s
80470016/221551911 [=========>....................] - ETA: 14s
80961536/221551911 [=========>....................] - ETA: 14s
81453056/221551911 [==========>...................] - ETA: 14s
81960960/221551911 [==========>...................] - ETA: 14s
82468864/221551911 [==========>...................] - ETA: 14s
82993152/221551911 [==========>...................] - ETA: 14s
83517440/221551911 [==========>...................] - ETA: 14s
84041728/221551911 [==========>...................] - ETA: 14s
84566016/221551911 [==========>...................] - ETA: 13s
85106688/221551911 [==========>...................] - ETA: 13s
85630976/221551911 [==========>...................] - ETA: 13s
86155264/221551911 [==========>...................] - ETA: 13s
86695936/221551911 [==========>...................] - ETA: 13s
87252992/221551911 [==========>...................] - ETA: 13s
87793664/221551911 [==========>...................] - ETA: 13s
88334336/221551911 [==========>...................] - ETA: 13s
88891392/221551911 [===========>..................] - ETA: 13s
89448448/221551911 [===========>..................] - ETA: 13s
89989120/221551911 [===========>..................] - ETA: 13s
90529792/221551911 [===========>..................] - ETA: 13s
91103232/221551911 [===========>..................] - ETA: 13s
91660288/221551911 [===========>..................] - ETA: 13s
92217344/221551911 [===========>..................] - ETA: 13s
92774400/221551911 [===========>..................] - ETA: 13s
93347840/221551911 [===========>..................] - ETA: 12s
93921280/221551911 [===========>..................] - ETA: 12s
94494720/221551911 [===========>..................] - ETA: 12s
95051776/221551911 [===========>..................] - ETA: 12s
95608832/221551911 [===========>..................] - ETA: 12s
96182272/221551911 [============>.................] - ETA: 12s
96739328/221551911 [============>.................] - ETA: 12s
97312768/221551911 [============>.................] - ETA: 12s
97886208/221551911 [============>.................] - ETA: 12s
98459648/221551911 [============>.................] - ETA: 12s
99033088/221551911 [============>.................] - ETA: 12s
99590144/221551911 [============>.................] - ETA: 12s
100147200/221551911 [============>.................] - ETA: 12s
100737024/221551911 [============>.................] - ETA: 12s
101310464/221551911 [============>.................] - ETA: 12s
101867520/221551911 [============>.................] - ETA: 11s
102440960/221551911 [============>.................] - ETA: 11s
102998016/221551911 [============>.................] - ETA: 11s
103555072/221551911 [=============>................] - ETA: 11s
104030208/221551911 [=============>................] - ETA: 11s
104488960/221551911 [=============>................] - ETA: 11s
104996864/221551911 [=============>................] - ETA: 11s
105472000/221551911 [=============>................] - ETA: 11s
105963520/221551911 [=============>................] - ETA: 11s
106471424/221551911 [=============>................] - ETA: 11s
106979328/221551911 [=============>................] - ETA: 11s
107503616/221551911 [=============>................] - ETA: 11s
108027904/221551911 [=============>................] - ETA: 11s
108535808/221551911 [=============>................] - ETA: 11s
109076480/221551911 [=============>................] - ETA: 11s
109600768/221551911 [=============>................] - ETA: 11s
110141440/221551911 [=============>................] - ETA: 11s
110682112/221551911 [=============>................] - ETA: 11s
111222784/221551911 [==============>...............] - ETA: 11s
111763456/221551911 [==============>...............] - ETA: 10s
112320512/221551911 [==============>...............] - ETA: 10s
112877568/221551911 [==============>...............] - ETA: 10s
113401856/221551911 [==============>...............] - ETA: 10s
113909760/221551911 [==============>...............] - ETA: 10s
114188288/221551911 [==============>...............] - ETA: 10s
114696192/221551911 [==============>...............] - ETA: 10s
115130368/221551911 [==============>...............] - ETA: 10s
115367936/221551911 [==============>...............] - ETA: 10s
115417088/221551911 [==============>...............] - ETA: 10s
115761152/221551911 [==============>...............] - ETA: 10s
115908608/221551911 [==============>...............] - ETA: 10s
116056064/221551911 [==============>...............] - ETA: 10s
116203520/221551911 [==============>...............] - ETA: 10s
116350976/221551911 [==============>...............] - ETA: 10s
116498432/221551911 [==============>...............] - ETA: 10s
116645888/221551911 [==============>...............] - ETA: 10s
116727808/221551911 [==============>...............] - ETA: 10s
116875264/221551911 [==============>...............] - ETA: 10s
116940800/221551911 [==============>...............] - ETA: 10s
117022720/221551911 [==============>...............] - ETA: 10s
117121024/221551911 [==============>...............] - ETA: 11s
117153792/221551911 [==============>...............] - ETA: 11s
117186560/221551911 [==============>...............] - ETA: 11s
117235712/221551911 [==============>...............] - ETA: 11s
117301248/221551911 [==============>...............] - ETA: 11s
117366784/221551911 [==============>...............] - ETA: 11s
117432320/221551911 [==============>...............] - ETA: 11s
117514240/221551911 [==============>...............] - ETA: 11s
117596160/221551911 [==============>...............] - ETA: 11s
117645312/221551911 [==============>...............] - ETA: 11s
117710848/221551911 [==============>...............] - ETA: 11s
117760000/221551911 [==============>...............] - ETA: 11s
117809152/221551911 [==============>...............] - ETA: 11s
117874688/221551911 [==============>...............] - ETA: 11s
117940224/221551911 [==============>...............] - ETA: 11s
118005760/221551911 [==============>...............] - ETA: 11s
118087680/221551911 [==============>...............] - ETA: 11s
118169600/221551911 [===============>..............] - ETA: 11s
118267904/221551911 [===============>..............] - ETA: 11s
118366208/221551911 [===============>..............] - ETA: 11s
118448128/221551911 [===============>..............] - ETA: 11s
118546432/221551911 [===============>..............] - ETA: 11s
118644736/221551911 [===============>..............] - ETA: 11s
118743040/221551911 [===============>..............] - ETA: 11s
118857728/221551911 [===============>..............] - ETA: 11s
118939648/221551911 [===============>..............] - ETA: 11s
119054336/221551911 [===============>..............] - ETA: 11s
119136256/221551911 [===============>..............] - ETA: 11s
119234560/221551911 [===============>..............] - ETA: 11s
119316480/221551911 [===============>..............] - ETA: 12s
119365632/221551911 [===============>..............] - ETA: 12s
119447552/221551911 [===============>..............] - ETA: 12s
119529472/221551911 [===============>..............] - ETA: 12s
119611392/221551911 [===============>..............] - ETA: 12s
119676928/221551911 [===============>..............] - ETA: 12s
119742464/221551911 [===============>..............] - ETA: 12s
119824384/221551911 [===============>..............] - ETA: 12s
119922688/221551911 [===============>..............] - ETA: 12s
119988224/221551911 [===============>..............] - ETA: 12s
120053760/221551911 [===============>..............] - ETA: 12s
120119296/221551911 [===============>..............] - ETA: 12s
120201216/221551911 [===============>..............] - ETA: 12s
120266752/221551911 [===============>..............] - ETA: 12s
120315904/221551911 [===============>..............] - ETA: 12s
120397824/221551911 [===============>..............] - ETA: 12s
120479744/221551911 [===============>..............] - ETA: 12s
120545280/221551911 [===============>..............] - ETA: 12s
120692736/221551911 [===============>..............] - ETA: 12s
120856576/221551911 [===============>..............] - ETA: 12s
121053184/221551911 [===============>..............] - ETA: 12s
121233408/221551911 [===============>..............] - ETA: 12s
121430016/221551911 [===============>..............] - ETA: 12s
121626624/221551911 [===============>..............] - ETA: 12s
121823232/221551911 [===============>..............] - ETA: 12s
122036224/221551911 [===============>..............] - ETA: 12s
122232832/221551911 [===============>..............] - ETA: 12s
122462208/221551911 [===============>..............] - ETA: 12s
122658816/221551911 [===============>..............] - ETA: 12s
122888192/221551911 [===============>..............] - ETA: 12s
123101184/221551911 [===============>..............] - ETA: 12s
123330560/221551911 [===============>..............] - ETA: 12s
123559936/221551911 [===============>..............] - ETA: 12s
123789312/221551911 [===============>..............] - ETA: 12s
124043264/221551911 [===============>..............] - ETA: 12s
124297216/221551911 [===============>..............] - ETA: 12s
124559360/221551911 [===============>..............] - ETA: 12s
124837888/221551911 [===============>..............] - ETA: 12s
125083648/221551911 [===============>..............] - ETA: 12s
125362176/221551911 [===============>..............] - ETA: 12s
125640704/221551911 [================>.............] - ETA: 12s
125919232/221551911 [================>.............] - ETA: 12s
126214144/221551911 [================>.............] - ETA: 12s
126509056/221551911 [================>.............] - ETA: 12s
126803968/221551911 [================>.............] - ETA: 12s
127098880/221551911 [================>.............] - ETA: 12s
127410176/221551911 [================>.............] - ETA: 12s
127721472/221551911 [================>.............] - ETA: 12s
128049152/221551911 [================>.............] - ETA: 12s
128376832/221551911 [================>.............] - ETA: 12s
128688128/221551911 [================>.............] - ETA: 12s
129032192/221551911 [================>.............] - ETA: 12s
129359872/221551911 [================>.............] - ETA: 11s
129703936/221551911 [================>.............] - ETA: 11s
130048000/221551911 [================>.............] - ETA: 11s
130392064/221551911 [================>.............] - ETA: 11s
130719744/221551911 [================>.............] - ETA: 11s
131096576/221551911 [================>.............] - ETA: 11s
131440640/221551911 [================>.............] - ETA: 11s
131801088/221551911 [================>.............] - ETA: 11s
132177920/221551911 [================>.............] - ETA: 11s
132571136/221551911 [================>.............] - ETA: 11s
132931584/221551911 [=================>............] - ETA: 11s
133324800/221551911 [=================>............] - ETA: 11s
133701632/221551911 [=================>............] - ETA: 11s
134127616/221551911 [=================>............] - ETA: 11s
134520832/221551911 [=================>............] - ETA: 11s
134930432/221551911 [=================>............] - ETA: 11s
135331840/221551911 [=================>............] - ETA: 11s
135749632/221551911 [=================>............] - ETA: 11s
136159232/221551911 [=================>............] - ETA: 11s
136568832/221551911 [=================>............] - ETA: 11s
136994816/221551911 [=================>............] - ETA: 11s
137420800/221551911 [=================>............] - ETA: 10s
137863168/221551911 [=================>............] - ETA: 10s
138272768/221551911 [=================>............] - ETA: 10s
138698752/221551911 [=================>............] - ETA: 10s
139141120/221551911 [=================>............] - ETA: 10s
139583488/221551911 [=================>............] - ETA: 10s
140025856/221551911 [=================>............] - ETA: 10s
140484608/221551911 [==================>...........] - ETA: 10s
140943360/221551911 [==================>...........] - ETA: 10s
141418496/221551911 [==================>...........] - ETA: 10s
141877248/221551911 [==================>...........] - ETA: 10s
142352384/221551911 [==================>...........] - ETA: 10s
142811136/221551911 [==================>...........] - ETA: 10s
143286272/221551911 [==================>...........] - ETA: 10s
143777792/221551911 [==================>...........] - ETA: 10s
144252928/221551911 [==================>...........] - ETA: 10s
144744448/221551911 [==================>...........] - ETA: 9s
145219584/221551911 [==================>...........] - ETA: 9s
145711104/221551911 [==================>...........] - ETA: 9s
146202624/221551911 [==================>...........] - ETA: 9s
146710528/221551911 [==================>...........] - ETA: 9s
147218432/221551911 [==================>...........] - ETA: 9s
147709952/221551911 [===================>..........] - ETA: 9s
148217856/221551911 [===================>..........] - ETA: 9s
148725760/221551911 [===================>..........] - ETA: 9s
149233664/221551911 [===================>..........] - ETA: 9s
149774336/221551911 [===================>..........] - ETA: 9s
150298624/221551911 [===================>..........] - ETA: 9s
150822912/221551911 [===================>..........] - ETA: 9s
151347200/221551911 [===================>..........] - ETA: 8s
151887872/221551911 [===================>..........] - ETA: 8s
152444928/221551911 [===================>..........] - ETA: 8s
152985600/221551911 [===================>..........] - ETA: 8s
153526272/221551911 [===================>..........] - ETA: 8s
154083328/221551911 [===================>..........] - ETA: 8s
154624000/221551911 [===================>..........] - ETA: 8s
155131904/221551911 [====================>.........] - ETA: 8s
155607040/221551911 [====================>.........] - ETA: 8s
156016640/221551911 [====================>.........] - ETA: 8s
156442624/221551911 [====================>.........] - ETA: 8s
156868608/221551911 [====================>.........] - ETA: 8s
157278208/221551911 [====================>.........] - ETA: 8s
157720576/221551911 [====================>.........] - ETA: 8s
158162944/221551911 [====================>.........] - ETA: 8s
158507008/221551911 [====================>.........] - ETA: 8s
158998528/221551911 [====================>.........] - ETA: 7s
159326208/221551911 [====================>.........] - ETA: 7s
159637504/221551911 [====================>.........] - ETA: 7s
159965184/221551911 [====================>.........] - ETA: 7s
160292864/221551911 [====================>.........] - ETA: 7s
160636928/221551911 [====================>.........] - ETA: 7s
160980992/221551911 [====================>.........] - ETA: 7s
161325056/221551911 [====================>.........] - ETA: 7s
161669120/221551911 [====================>.........] - ETA: 7s
162029568/221551911 [====================>.........] - ETA: 7s
162406400/221551911 [====================>.........] - ETA: 7s
162750464/221551911 [=====================>........] - ETA: 7s
163127296/221551911 [=====================>........] - ETA: 7s
163471360/221551911 [=====================>........] - ETA: 7s
163618816/221551911 [=====================>........] - ETA: 7s
163799040/221551911 [=====================>........] - ETA: 7s
164077568/221551911 [=====================>........] - ETA: 7s
164225024/221551911 [=====================>........] - ETA: 7s
164356096/221551911 [=====================>........] - ETA: 7s
164503552/221551911 [=====================>........] - ETA: 7s
164667392/221551911 [=====================>........] - ETA: 7s
164831232/221551911 [=====================>........] - ETA: 7s
164978688/221551911 [=====================>........] - ETA: 7s
165142528/221551911 [=====================>........] - ETA: 7s
165306368/221551911 [=====================>........] - ETA: 7s
165486592/221551911 [=====================>........] - ETA: 7s
165666816/221551911 [=====================>........] - ETA: 7s
165781504/221551911 [=====================>........] - ETA: 7s
165879808/221551911 [=====================>........] - ETA: 7s
166010880/221551911 [=====================>........] - ETA: 7s
166125568/221551911 [=====================>........] - ETA: 7s
166223872/221551911 [=====================>........] - ETA: 7s
166338560/221551911 [=====================>........] - ETA: 7s
166453248/221551911 [=====================>........] - ETA: 7s
166567936/221551911 [=====================>........] - ETA: 7s
166666240/221551911 [=====================>........] - ETA: 7s
166731776/221551911 [=====================>........] - ETA: 7s
166813696/221551911 [=====================>........] - ETA: 7s
166895616/221551911 [=====================>........] - ETA: 7s
166977536/221551911 [=====================>........] - ETA: 7s
167059456/221551911 [=====================>........] - ETA: 7s
167157760/221551911 [=====================>........] - ETA: 7s
167256064/221551911 [=====================>........] - ETA: 7s
167337984/221551911 [=====================>........] - ETA: 7s
167403520/221551911 [=====================>........] - ETA: 7s
167452672/221551911 [=====================>........] - ETA: 7s
167501824/221551911 [=====================>........] - ETA: 7s
167550976/221551911 [=====================>........] - ETA: 7s
167600128/221551911 [=====================>........] - ETA: 7s
167649280/221551911 [=====================>........] - ETA: 7s
167698432/221551911 [=====================>........] - ETA: 7s
167763968/221551911 [=====================>........] - ETA: 7s
167813120/221551911 [=====================>........] - ETA: 7s
167878656/221551911 [=====================>........] - ETA: 7s
167927808/221551911 [=====================>........] - ETA: 7s
167993344/221551911 [=====================>........] - ETA: 7s
168058880/221551911 [=====================>........] - ETA: 7s
168124416/221551911 [=====================>........] - ETA: 7s
168189952/221551911 [=====================>........] - ETA: 7s
168271872/221551911 [=====================>........] - ETA: 7s
168353792/221551911 [=====================>........] - ETA: 7s
168435712/221551911 [=====================>........] - ETA: 7s
168534016/221551911 [=====================>........] - ETA: 7s
168599552/221551911 [=====================>........] - ETA: 7s
168648704/221551911 [=====================>........] - ETA: 7s
168665088/221551911 [=====================>........] - ETA: 7s
168730624/221551911 [=====================>........] - ETA: 7s
168779776/221551911 [=====================>........] - ETA: 7s
168812544/221551911 [=====================>........] - ETA: 7s
168845312/221551911 [=====================>........] - ETA: 7s
168894464/221551911 [=====================>........] - ETA: 7s
168960000/221551911 [=====================>........] - ETA: 7s
169025536/221551911 [=====================>........] - ETA: 7s
169091072/221551911 [=====================>........] - ETA: 7s
169123840/221551911 [=====================>........] - ETA: 7s
169189376/221551911 [=====================>........] - ETA: 7s
169238528/221551911 [=====================>........] - ETA: 7s
169287680/221551911 [=====================>........] - ETA: 7s
169336832/221551911 [=====================>........] - ETA: 7s
169369600/221551911 [=====================>........] - ETA: 7s
169418752/221551911 [=====================>........] - ETA: 7s
169435136/221551911 [=====================>........] - ETA: 7s
169451520/221551911 [=====================>........] - ETA: 7s
169484288/221551911 [=====================>........] - ETA: 7s
169517056/221551911 [=====================>........] - ETA: 7s
169549824/221551911 [=====================>........] - ETA: 7s
169582592/221551911 [=====================>........] - ETA: 7s
169631744/221551911 [=====================>........] - ETA: 7s
169664512/221551911 [=====================>........] - ETA: 7s
169697280/221551911 [=====================>........] - ETA: 7s
169730048/221551911 [=====================>........] - ETA: 7s
169762816/221551911 [=====================>........] - ETA: 7s
169795584/221551911 [=====================>........] - ETA: 7s
169844736/221551911 [=====================>........] - ETA: 7s
169893888/221551911 [======================>.......] - ETA: 7s
169943040/221551911 [======================>.......] - ETA: 7s
170008576/221551911 [======================>.......] - ETA: 7s
170074112/221551911 [======================>.......] - ETA: 7s
170139648/221551911 [======================>.......] - ETA: 7s
170221568/221551911 [======================>.......] - ETA: 7s
170303488/221551911 [======================>.......] - ETA: 7s
170319872/221551911 [======================>.......] - ETA: 7s
170385408/221551911 [======================>.......] - ETA: 7s
170450944/221551911 [======================>.......] - ETA: 7s
170516480/221551911 [======================>.......] - ETA: 8s
170582016/221551911 [======================>.......] - ETA: 8s
170647552/221551911 [======================>.......] - ETA: 8s
170729472/221551911 [======================>.......] - ETA: 8s
170778624/221551911 [======================>.......] - ETA: 8s
170844160/221551911 [======================>.......] - ETA: 8s
170893312/221551911 [======================>.......] - ETA: 8s
170926080/221551911 [======================>.......] - ETA: 8s
170975232/221551911 [======================>.......] - ETA: 8s
171024384/221551911 [======================>.......] - ETA: 8s
171073536/221551911 [======================>.......] - ETA: 8s
171122688/221551911 [======================>.......] - ETA: 8s
171188224/221551911 [======================>.......] - ETA: 8s
171253760/221551911 [======================>.......] - ETA: 8s
171335680/221551911 [======================>.......] - ETA: 8s
171384832/221551911 [======================>.......] - ETA: 8s
171450368/221551911 [======================>.......] - ETA: 8s
171515904/221551911 [======================>.......] - ETA: 8s
171581440/221551911 [======================>.......] - ETA: 8s
171646976/221551911 [======================>.......] - ETA: 8s
171728896/221551911 [======================>.......] - ETA: 8s
171810816/221551911 [======================>.......] - ETA: 8s
171892736/221551911 [======================>.......] - ETA: 8s
171974656/221551911 [======================>.......] - ETA: 8s
172023808/221551911 [======================>.......] - ETA: 8s
172122112/221551911 [======================>.......] - ETA: 8s
172187648/221551911 [======================>.......] - ETA: 8s
172269568/221551911 [======================>.......] - ETA: 8s
172351488/221551911 [======================>.......] - ETA: 8s
172417024/221551911 [======================>.......] - ETA: 8s
172482560/221551911 [======================>.......] - ETA: 8s
172548096/221551911 [======================>.......] - ETA: 8s
172597248/221551911 [======================>.......] - ETA: 8s
172646400/221551911 [======================>.......] - ETA: 8s
172711936/221551911 [======================>.......] - ETA: 8s
172761088/221551911 [======================>.......] - ETA: 8s
172793856/221551911 [======================>.......] - ETA: 8s
172843008/221551911 [======================>.......] - ETA: 8s
172875776/221551911 [======================>.......] - ETA: 8s
172908544/221551911 [======================>.......] - ETA: 8s
172941312/221551911 [======================>.......] - ETA: 8s
172974080/221551911 [======================>.......] - ETA: 8s
173006848/221551911 [======================>.......] - ETA: 8s
173023232/221551911 [======================>.......] - ETA: 8s
173072384/221551911 [======================>.......] - ETA: 8s
173105152/221551911 [======================>.......] - ETA: 8s
173137920/221551911 [======================>.......] - ETA: 8s
173187072/221551911 [======================>.......] - ETA: 8s
173252608/221551911 [======================>.......] - ETA: 8s
173318144/221551911 [======================>.......] - ETA: 8s
173383680/221551911 [======================>.......] - ETA: 8s
173449216/221551911 [======================>.......] - ETA: 8s
173531136/221551911 [======================>.......] - ETA: 8s
173613056/221551911 [======================>.......] - ETA: 8s
173678592/221551911 [======================>.......] - ETA: 8s
173727744/221551911 [======================>.......] - ETA: 8s
173776896/221551911 [======================>.......] - ETA: 8s
173842432/221551911 [======================>.......] - ETA: 8s
173875200/221551911 [======================>.......] - ETA: 8s
173924352/221551911 [======================>.......] - ETA: 8s
173973504/221551911 [======================>.......] - ETA: 8s
174039040/221551911 [======================>.......] - ETA: 8s
174104576/221551911 [======================>.......] - ETA: 8s
174170112/221551911 [======================>.......] - ETA: 8s
174235648/221551911 [======================>.......] - ETA: 8s
174301184/221551911 [======================>.......] - ETA: 8s
174383104/221551911 [======================>.......] - ETA: 8s
174465024/221551911 [======================>.......] - ETA: 8s
174563328/221551911 [======================>.......] - ETA: 8s
174645248/221551911 [======================>.......] - ETA: 8s
174743552/221551911 [======================>.......] - ETA: 8s
174809088/221551911 [======================>.......] - ETA: 8s
174874624/221551911 [======================>.......] - ETA: 8s
174940160/221551911 [======================>.......] - ETA: 8s
175005696/221551911 [======================>.......] - ETA: 8s
175054848/221551911 [======================>.......] - ETA: 8s
175136768/221551911 [======================>.......] - ETA: 8s
175218688/221551911 [======================>.......] - ETA: 8s
175284224/221551911 [======================>.......] - ETA: 8s
175382528/221551911 [======================>.......] - ETA: 8s
175480832/221551911 [======================>.......] - ETA: 8s
175529984/221551911 [======================>.......] - ETA: 8s
175595520/221551911 [======================>.......] - ETA: 8s
175644672/221551911 [======================>.......] - ETA: 8s
175693824/221551911 [======================>.......] - ETA: 8s
175759360/221551911 [======================>.......] - ETA: 8s
175824896/221551911 [======================>.......] - ETA: 8s
175906816/221551911 [======================>.......] - ETA: 8s
175972352/221551911 [======================>.......] - ETA: 8s
176054272/221551911 [======================>.......] - ETA: 8s
176119808/221551911 [======================>.......] - ETA: 8s
176168960/221551911 [======================>.......] - ETA: 8s
176250880/221551911 [======================>.......] - ETA: 8s
176300032/221551911 [======================>.......] - ETA: 8s
176349184/221551911 [======================>.......] - ETA: 8s
176398336/221551911 [======================>.......] - ETA: 8s
176447488/221551911 [======================>.......] - ETA: 8s
176496640/221551911 [======================>.......] - ETA: 8s
176545792/221551911 [======================>.......] - ETA: 8s
176578560/221551911 [======================>.......] - ETA: 8s
176627712/221551911 [======================>.......] - ETA: 8s
176676864/221551911 [======================>.......] - ETA: 8s
176709632/221551911 [======================>.......] - ETA: 8s
176758784/221551911 [======================>.......] - ETA: 8s
176791552/221551911 [======================>.......] - ETA: 8s
176824320/221551911 [======================>.......] - ETA: 8s
176857088/221551911 [======================>.......] - ETA: 8s
176889856/221551911 [======================>.......] - ETA: 8s
176939008/221551911 [======================>.......] - ETA: 8s
176971776/221551911 [======================>.......] - ETA: 8s
177020928/221551911 [======================>.......] - ETA: 8s
177070080/221551911 [======================>.......] - ETA: 8s
177119232/221551911 [======================>.......] - ETA: 8s
177184768/221551911 [======================>.......] - ETA: 8s
177250304/221551911 [=======================>......] - ETA: 8s
177315840/221551911 [=======================>......] - ETA: 8s
177397760/221551911 [=======================>......] - ETA: 8s
177479680/221551911 [=======================>......] - ETA: 8s
177577984/221551911 [=======================>......] - ETA: 8s
177676288/221551911 [=======================>......] - ETA: 8s
177774592/221551911 [=======================>......] - ETA: 8s
177872896/221551911 [=======================>......] - ETA: 8s
177987584/221551911 [=======================>......] - ETA: 8s
178102272/221551911 [=======================>......] - ETA: 8s
178216960/221551911 [=======================>......] - ETA: 8s
178348032/221551911 [=======================>......] - ETA: 8s
178479104/221551911 [=======================>......] - ETA: 8s
178610176/221551911 [=======================>......] - ETA: 8s
178741248/221551911 [=======================>......] - ETA: 8s
178888704/221551911 [=======================>......] - ETA: 8s
179036160/221551911 [=======================>......] - ETA: 8s
179183616/221551911 [=======================>......] - ETA: 8s
179347456/221551911 [=======================>......] - ETA: 8s
179511296/221551911 [=======================>......] - ETA: 8s
179675136/221551911 [=======================>......] - ETA: 8s
179838976/221551911 [=======================>......] - ETA: 8s
180019200/221551911 [=======================>......] - ETA: 8s
180133888/221551911 [=======================>......] - ETA: 8s
180314112/221551911 [=======================>......] - ETA: 8s
180453376/221551911 [=======================>......] - ETA: 7s
180576256/221551911 [=======================>......] - ETA: 7s
180658176/221551911 [=======================>......] - ETA: 7s
180805632/221551911 [=======================>......] - ETA: 7s
180903936/221551911 [=======================>......] - ETA: 7s
181018624/221551911 [=======================>......] - ETA: 7s
181100544/221551911 [=======================>......] - ETA: 7s
181182464/221551911 [=======================>......] - ETA: 7s
181280768/221551911 [=======================>......] - ETA: 7s
181362688/221551911 [=======================>......] - ETA: 7s
181460992/221551911 [=======================>......] - ETA: 7s
181559296/221551911 [=======================>......] - ETA: 7s
181641216/221551911 [=======================>......] - ETA: 7s
181690368/221551911 [=======================>......] - ETA: 7s
181788672/221551911 [=======================>......] - ETA: 7s
181870592/221551911 [=======================>......] - ETA: 7s
181952512/221551911 [=======================>......] - ETA: 7s
182050816/221551911 [=======================>......] - ETA: 7s
182116352/221551911 [=======================>......] - ETA: 7s
182181888/221551911 [=======================>......] - ETA: 7s
182214656/221551911 [=======================>......] - ETA: 7s
182296576/221551911 [=======================>......] - ETA: 7s
182345728/221551911 [=======================>......] - ETA: 7s
182411264/221551911 [=======================>......] - ETA: 7s
182493184/221551911 [=======================>......] - ETA: 7s
182558720/221551911 [=======================>......] - ETA: 7s
182607872/221551911 [=======================>......] - ETA: 7s
182657024/221551911 [=======================>......] - ETA: 7s
182706176/221551911 [=======================>......] - ETA: 7s
182755328/221551911 [=======================>......] - ETA: 7s
182820864/221551911 [=======================>......] - ETA: 7s
182870016/221551911 [=======================>......] - ETA: 7s
182935552/221551911 [=======================>......] - ETA: 7s
183017472/221551911 [=======================>......] - ETA: 7s
183099392/221551911 [=======================>......] - ETA: 7s
183181312/221551911 [=======================>......] - ETA: 7s
183263232/221551911 [=======================>......] - ETA: 7s
183345152/221551911 [=======================>......] - ETA: 7s
183443456/221551911 [=======================>......] - ETA: 7s
183558144/221551911 [=======================>......] - ETA: 7s
183672832/221551911 [=======================>......] - ETA: 7s
183705600/221551911 [=======================>......] - ETA: 7s
183787520/221551911 [=======================>......] - ETA: 7s
183869440/221551911 [=======================>......] - ETA: 7s
183934976/221551911 [=======================>......] - ETA: 7s
184000512/221551911 [=======================>......] - ETA: 7s
184082432/221551911 [=======================>......] - ETA: 7s
184147968/221551911 [=======================>......] - ETA: 7s
184229888/221551911 [=======================>......] - ETA: 7s
184311808/221551911 [=======================>......] - ETA: 7s
184393728/221551911 [=======================>......] - ETA: 7s
184442880/221551911 [=======================>......] - ETA: 7s
184541184/221551911 [=======================>......] - ETA: 7s
184623104/221551911 [=======================>......] - ETA: 7s
184688640/221551911 [========================>.....] - ETA: 7s
184754176/221551911 [========================>.....] - ETA: 7s
184819712/221551911 [========================>.....] - ETA: 7s
184868864/221551911 [========================>.....] - ETA: 7s
184918016/221551911 [========================>.....] - ETA: 7s
184999936/221551911 [========================>.....] - ETA: 7s
185049088/221551911 [========================>.....] - ETA: 7s
185098240/221551911 [========================>.....] - ETA: 7s
185147392/221551911 [========================>.....] - ETA: 7s
185196544/221551911 [========================>.....] - ETA: 7s
185245696/221551911 [========================>.....] - ETA: 7s
185311232/221551911 [========================>.....] - ETA: 7s
185360384/221551911 [========================>.....] - ETA: 7s
185409536/221551911 [========================>.....] - ETA: 7s
185475072/221551911 [========================>.....] - ETA: 7s
185507840/221551911 [========================>.....] - ETA: 7s
185589760/221551911 [========================>.....] - ETA: 7s
185638912/221551911 [========================>.....] - ETA: 7s
185696256/221551911 [========================>.....] - ETA: 7s
185753600/221551911 [========================>.....] - ETA: 7s
185819136/221551911 [========================>.....] - ETA: 7s
185901056/221551911 [========================>.....] - ETA: 7s
185966592/221551911 [========================>.....] - ETA: 7s
186056704/221551911 [========================>.....] - ETA: 7s
186130432/221551911 [========================>.....] - ETA: 7s
186212352/221551911 [========================>.....] - ETA: 7s
186294272/221551911 [========================>.....] - ETA: 7s
186376192/221551911 [========================>.....] - ETA: 7s
186458112/221551911 [========================>.....] - ETA: 7s
186540032/221551911 [========================>.....] - ETA: 7s
186605568/221551911 [========================>.....] - ETA: 7s
186671104/221551911 [========================>.....] - ETA: 7s
186736640/221551911 [========================>.....] - ETA: 7s
186818560/221551911 [========================>.....] - ETA: 7s
186884096/221551911 [========================>.....] - ETA: 7s
186949632/221551911 [========================>.....] - ETA: 7s
186982400/221551911 [========================>.....] - ETA: 7s
187064320/221551911 [========================>.....] - ETA: 7s
187129856/221551911 [========================>.....] - ETA: 7s
187195392/221551911 [========================>.....] - ETA: 7s
187260928/221551911 [========================>.....] - ETA: 7s
187342848/221551911 [========================>.....] - ETA: 7s
187424768/221551911 [========================>.....] - ETA: 7s
187523072/221551911 [========================>.....] - ETA: 7s
187621376/221551911 [========================>.....] - ETA: 7s
187703296/221551911 [========================>.....] - ETA: 7s
187801600/221551911 [========================>.....] - ETA: 7s
187916288/221551911 [========================>.....] - ETA: 7s
188047360/221551911 [========================>.....] - ETA: 7s
188178432/221551911 [========================>.....] - ETA: 7s
188309504/221551911 [========================>.....] - ETA: 7s
188456960/221551911 [========================>.....] - ETA: 7s
188604416/221551911 [========================>.....] - ETA: 7s
188751872/221551911 [========================>.....] - ETA: 7s
188882944/221551911 [========================>.....] - ETA: 7s
189030400/221551911 [========================>.....] - ETA: 7s
189210624/221551911 [========================>.....] - ETA: 7s
189390848/221551911 [========================>.....] - ETA: 7s
189587456/221551911 [========================>.....] - ETA: 7s
189767680/221551911 [========================>.....] - ETA: 7s
189980672/221551911 [========================>.....] - ETA: 6s
190193664/221551911 [========================>.....] - ETA: 6s
190406656/221551911 [========================>.....] - ETA: 6s
190619648/221551911 [========================>.....] - ETA: 6s
190849024/221551911 [========================>.....] - ETA: 6s
191078400/221551911 [========================>.....] - ETA: 6s
191307776/221551911 [========================>.....] - ETA: 6s
191488000/221551911 [========================>.....] - ETA: 6s
191733760/221551911 [========================>.....] - ETA: 6s
191979520/221551911 [========================>.....] - ETA: 6s
192176128/221551911 [=========================>....] - ETA: 6s
192421888/221551911 [=========================>....] - ETA: 6s
192667648/221551911 [=========================>....] - ETA: 6s
192897024/221551911 [=========================>....] - ETA: 6s
193159168/221551911 [=========================>....] - ETA: 6s
193388544/221551911 [=========================>....] - ETA: 6s
193667072/221551911 [=========================>....] - ETA: 6s
193912832/221551911 [=========================>....] - ETA: 6s
194174976/221551911 [=========================>....] - ETA: 6s
194437120/221551911 [=========================>....] - ETA: 5s
194715648/221551911 [=========================>....] - ETA: 5s
194994176/221551911 [=========================>....] - ETA: 5s
195289088/221551911 [=========================>....] - ETA: 5s
195567616/221551911 [=========================>....] - ETA: 5s
195862528/221551911 [=========================>....] - ETA: 5s
196141056/221551911 [=========================>....] - ETA: 5s
196468736/221551911 [=========================>....] - ETA: 5s
196747264/221551911 [=========================>....] - ETA: 5s
197074944/221551911 [=========================>....] - ETA: 5s
197402624/221551911 [=========================>....] - ETA: 5s
197713920/221551911 [=========================>....] - ETA: 5s
198057984/221551911 [=========================>....] - ETA: 5s
198369280/221551911 [=========================>....] - ETA: 5s
198713344/221551911 [=========================>....] - ETA: 5s
199041024/221551911 [=========================>....] - ETA: 4s
199385088/221551911 [=========================>....] - ETA: 4s
199745536/221551911 [==========================>...] - ETA: 4s
200089600/221551911 [==========================>...] - ETA: 4s
200466432/221551911 [==========================>...] - ETA: 4s
200810496/221551911 [==========================>...] - ETA: 4s
201203712/221551911 [==========================>...] - ETA: 4s
201547776/221551911 [==========================>...] - ETA: 4s
201940992/221551911 [==========================>...] - ETA: 4s
202301440/221551911 [==========================>...] - ETA: 4s
202711040/221551911 [==========================>...] - ETA: 4s
203120640/221551911 [==========================>...] - ETA: 4s
203497472/221551911 [==========================>...] - ETA: 3s
203907072/221551911 [==========================>...] - ETA: 3s
204283904/221551911 [==========================>...] - ETA: 3s
204709888/221551911 [==========================>...] - ETA: 3s
205103104/221551911 [==========================>...] - ETA: 3s
205545472/221551911 [==========================>...] - ETA: 3s
205955072/221551911 [==========================>...] - ETA: 3s
206381056/221551911 [==========================>...] - ETA: 3s
206823424/221551911 [===========================>..] - ETA: 3s
207249408/221551911 [===========================>..] - ETA: 3s
207691776/221551911 [===========================>..] - ETA: 2s
208134144/221551911 [===========================>..] - ETA: 2s
208592896/221551911 [===========================>..] - ETA: 2s
209051648/221551911 [===========================>..] - ETA: 2s
209510400/221551911 [===========================>..] - ETA: 2s
209969152/221551911 [===========================>..] - ETA: 2s
210444288/221551911 [===========================>..] - ETA: 2s
210919424/221551911 [===========================>..] - ETA: 2s
211410944/221551911 [===========================>..] - ETA: 2s
211886080/221551911 [===========================>..] - ETA: 2s
212377600/221551911 [===========================>..] - ETA: 1s
212869120/221551911 [===========================>..] - ETA: 1s
213360640/221551911 [===========================>..] - ETA: 1s
213835776/221551911 [===========================>..] - ETA: 1s
214343680/221551911 [============================>.] - ETA: 1s
214851584/221551911 [============================>.] - ETA: 1s
215375872/221551911 [============================>.] - ETA: 1s
215883776/221551911 [============================>.] - ETA: 1s
216408064/221551911 [============================>.] - ETA: 1s
216883200/221551911 [============================>.] - ETA: 0s
217407488/221551911 [============================>.] - ETA: 0s
217948160/221551911 [============================>.] - ETA: 0s
218488832/221551911 [============================>.] - ETA: 0s
219013120/221551911 [============================>.] - ETA: 0s
219553792/221551911 [============================>.] - ETA: 0s
220094464/221551911 [============================>.] - ETA: 0s
220635136/221551911 [============================>.] - ETA: 0s
221192192/221551911 [============================>.] - ETA: 0s
221551911/221551911 [==============================] - 46s 0us/step
Loaded VOC2007 test data for car and person classes: 2500 images.
Anchors can also be computed easily using YOLO toolkit.
Note
The following code is given as an example. In a real use case scenario, anchors are computed on the training dataset.
from akida_models.detection.generate_anchors import generate_anchors
num_anchors = 5
grid_size = (7, 7)
anchors_example = generate_anchors(val_data, num_anchors, grid_size)
Average IOU for 5 anchors: 0.62
Anchors: [[0.55528, 1.15726], [1.45361, 1.95377], [1.47976, 4.12346], [2.95301, 4.75658], [5.52358, 5.45771]]
3. Model architecture
The model zoo contains a YOLO model that is built upon the AkidaNet architecture and 3 separable convolutional layers at the top for bounding box and class estimation followed by a final separable convolutional which is the detection layer. Note that for efficiency, the alpha parameter in AkidaNet (network width or number of filter in each layer) is set to 0.5.
from akida_models import yolo_base
# Create a yolo model for 2 classes with 5 anchors and grid size of 7
classes = 2
model = yolo_base(input_shape=(224, 224, 3),
classes=classes,
nb_box=num_anchors,
alpha=0.5)
model.summary()
Model: "yolo_base"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
input (InputLayer) [(None, 224, 224, 3)] 0
rescaling (Rescaling) (None, 224, 224, 3) 0
conv_0 (Conv2D) (None, 112, 112, 16) 432
conv_0/BN (BatchNormalizati (None, 112, 112, 16) 64
on)
conv_0/relu (ReLU) (None, 112, 112, 16) 0
conv_1 (Conv2D) (None, 112, 112, 32) 4608
conv_1/BN (BatchNormalizati (None, 112, 112, 32) 128
on)
conv_1/relu (ReLU) (None, 112, 112, 32) 0
conv_2 (Conv2D) (None, 56, 56, 64) 18432
conv_2/BN (BatchNormalizati (None, 56, 56, 64) 256
on)
conv_2/relu (ReLU) (None, 56, 56, 64) 0
conv_3 (Conv2D) (None, 56, 56, 64) 36864
conv_3/BN (BatchNormalizati (None, 56, 56, 64) 256
on)
conv_3/relu (ReLU) (None, 56, 56, 64) 0
dw_separable_4 (DepthwiseCo (None, 28, 28, 64) 576
nv2D)
pw_separable_4 (Conv2D) (None, 28, 28, 128) 8192
pw_separable_4/BN (BatchNor (None, 28, 28, 128) 512
malization)
pw_separable_4/relu (ReLU) (None, 28, 28, 128) 0
dw_separable_5 (DepthwiseCo (None, 28, 28, 128) 1152
nv2D)
pw_separable_5 (Conv2D) (None, 28, 28, 128) 16384
pw_separable_5/BN (BatchNor (None, 28, 28, 128) 512
malization)
pw_separable_5/relu (ReLU) (None, 28, 28, 128) 0
dw_separable_6 (DepthwiseCo (None, 14, 14, 128) 1152
nv2D)
pw_separable_6 (Conv2D) (None, 14, 14, 256) 32768
pw_separable_6/BN (BatchNor (None, 14, 14, 256) 1024
malization)
pw_separable_6/relu (ReLU) (None, 14, 14, 256) 0
dw_separable_7 (DepthwiseCo (None, 14, 14, 256) 2304
nv2D)
pw_separable_7 (Conv2D) (None, 14, 14, 256) 65536
pw_separable_7/BN (BatchNor (None, 14, 14, 256) 1024
malization)
pw_separable_7/relu (ReLU) (None, 14, 14, 256) 0
dw_separable_8 (DepthwiseCo (None, 14, 14, 256) 2304
nv2D)
pw_separable_8 (Conv2D) (None, 14, 14, 256) 65536
pw_separable_8/BN (BatchNor (None, 14, 14, 256) 1024
malization)
pw_separable_8/relu (ReLU) (None, 14, 14, 256) 0
dw_separable_9 (DepthwiseCo (None, 14, 14, 256) 2304
nv2D)
pw_separable_9 (Conv2D) (None, 14, 14, 256) 65536
pw_separable_9/BN (BatchNor (None, 14, 14, 256) 1024
malization)
pw_separable_9/relu (ReLU) (None, 14, 14, 256) 0
dw_separable_10 (DepthwiseC (None, 14, 14, 256) 2304
onv2D)
pw_separable_10 (Conv2D) (None, 14, 14, 256) 65536
pw_separable_10/BN (BatchNo (None, 14, 14, 256) 1024
rmalization)
pw_separable_10/relu (ReLU) (None, 14, 14, 256) 0
dw_separable_11 (DepthwiseC (None, 14, 14, 256) 2304
onv2D)
pw_separable_11 (Conv2D) (None, 14, 14, 256) 65536
pw_separable_11/BN (BatchNo (None, 14, 14, 256) 1024
rmalization)
pw_separable_11/relu (ReLU) (None, 14, 14, 256) 0
dw_separable_12 (DepthwiseC (None, 7, 7, 256) 2304
onv2D)
pw_separable_12 (Conv2D) (None, 7, 7, 512) 131072
pw_separable_12/BN (BatchNo (None, 7, 7, 512) 2048
rmalization)
pw_separable_12/relu (ReLU) (None, 7, 7, 512) 0
dw_separable_13 (DepthwiseC (None, 7, 7, 512) 4608
onv2D)
pw_separable_13 (Conv2D) (None, 7, 7, 512) 262144
pw_separable_13/BN (BatchNo (None, 7, 7, 512) 2048
rmalization)
pw_separable_13/relu (ReLU) (None, 7, 7, 512) 0
dw_1conv (DepthwiseConv2D) (None, 7, 7, 512) 4608
pw_1conv (Conv2D) (None, 7, 7, 1024) 524288
pw_1conv/BN (BatchNormaliza (None, 7, 7, 1024) 4096
tion)
pw_1conv/relu (ReLU) (None, 7, 7, 1024) 0
dw_2conv (DepthwiseConv2D) (None, 7, 7, 1024) 9216
pw_2conv (Conv2D) (None, 7, 7, 1024) 1048576
pw_2conv/BN (BatchNormaliza (None, 7, 7, 1024) 4096
tion)
pw_2conv/relu (ReLU) (None, 7, 7, 1024) 0
dw_3conv (DepthwiseConv2D) (None, 7, 7, 1024) 9216
pw_3conv (Conv2D) (None, 7, 7, 1024) 1048576
pw_3conv/BN (BatchNormaliza (None, 7, 7, 1024) 4096
tion)
pw_3conv/relu (ReLU) (None, 7, 7, 1024) 0
dw_detection_layer (Depthwi (None, 7, 7, 1024) 9216
seConv2D)
pw_detection_layer (Conv2D) (None, 7, 7, 35) 35875
=================================================================
Total params: 3,573,715
Trainable params: 3,561,587
Non-trainable params: 12,128
_________________________________________________________________
The model output can be reshaped to a more natural shape of:
(grid_height, grid_width, anchors_box, 4 + 1 + num_classes)
where the “4 + 1” term represents the coordinates of the estimated bounding boxes (top left x, top left y, width and height) and a confidence score. In other words, the output channels are actually grouped by anchor boxes, and in each group one channel provides either a coordinate, a global confidence score or a class confidence score. This process is done automatically in the decode_output function.
from tensorflow.keras import Model
from tensorflow.keras.layers import Reshape
# Define a reshape output to be added to the YOLO model
output = Reshape((grid_size[1], grid_size[0], num_anchors, 4 + 1 + classes),
name="YOLO_output")(model.output)
# Build the complete model
full_model = Model(model.input, output)
full_model.output
<KerasTensor: shape=(None, 7, 7, 5, 7) dtype=float32 (created by layer 'YOLO_output')>
4. Training
As the YOLO model relies on Brainchip AkidaNet/ImageNet network, it is possible to perform transfer learning from ImageNet pretrained weights when training a YOLO model. See the PlantVillage transfer learning example for a detail explanation on transfer learning principles.
5. Performance
The model zoo also contains an helper method that allows to create a YOLO model for VOC and load pretrained weights for the car and person detection task and the corresponding anchors. The anchors are used to interpret the model outputs.
The metric used to evaluate YOLO is the mean average precision (mAP) which is the percentage of correct prediction and is given for an intersection over union (IoU) ratio. Scores in this example are given for the standard IoU of 0.5 meaning that a detection is considered valid if the intersection over union ratio with its ground truth equivalent is above 0.5.
Note
A call to evaluate_map will preprocess the images, make the call to
Model.predict
and use decode_output before computing precision for all classes.
from timeit import default_timer as timer
from akida_models import yolo_voc_pretrained
from akida_models.detection.map_evaluation import MapEvaluation
# Load the pretrained model along with anchors
model_keras, anchors = yolo_voc_pretrained()
model_keras.summary()
Downloading data from https://data.brainchip.com/dataset-mirror/voc/voc_anchors.pkl.
0/126 [..............................] - ETA: 0s
126/126 [==============================] - 0s 1us/step
Downloading data from https://data.brainchip.com/models/AkidaV2/yolo/yolo_akidanet_voc_i8_w4_a4.h5.
0/14557688 [..............................] - ETA: 0s
90112/14557688 [..............................] - ETA: 9s
327680/14557688 [..............................] - ETA: 4s
851968/14557688 [>.............................] - ETA: 2s
1228800/14557688 [=>............................] - ETA: 2s
1622016/14557688 [==>...........................] - ETA: 2s
2015232/14557688 [===>..........................] - ETA: 1s
2408448/14557688 [===>..........................] - ETA: 1s
2809856/14557688 [====>.........................] - ETA: 1s
3211264/14557688 [=====>........................] - ETA: 1s
3620864/14557688 [======>.......................] - ETA: 1s
4022272/14557688 [=======>......................] - ETA: 1s
4440064/14557688 [========>.....................] - ETA: 1s
4866048/14557688 [=========>....................] - ETA: 1s
5308416/14557688 [=========>....................] - ETA: 1s
5750784/14557688 [==========>...................] - ETA: 1s
6193152/14557688 [===========>..................] - ETA: 1s
6635520/14557688 [============>.................] - ETA: 1s
7077888/14557688 [=============>................] - ETA: 0s
7536640/14557688 [==============>...............] - ETA: 0s
7979008/14557688 [===============>..............] - ETA: 0s
8437760/14557688 [================>.............] - ETA: 0s
8896512/14557688 [=================>............] - ETA: 0s
9355264/14557688 [==================>...........] - ETA: 0s
9846784/14557688 [===================>..........] - ETA: 0s
10321920/14557688 [====================>.........] - ETA: 0s
10797056/14557688 [=====================>........] - ETA: 0s
11255808/14557688 [======================>.......] - ETA: 0s
11747328/14557688 [=======================>......] - ETA: 0s
12238848/14557688 [========================>.....] - ETA: 0s
12730368/14557688 [=========================>....] - ETA: 0s
13205504/14557688 [==========================>...] - ETA: 0s
13713408/14557688 [===========================>..] - ETA: 0s
14221312/14557688 [============================>.] - ETA: 0s
14557688/14557688 [==============================] - 2s 0us/step
Model: "model_1"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
input (InputLayer) [(None, 224, 224, 3)] 0
rescaling (QuantizedRescali (None, 224, 224, 3) 0
ng)
conv_0 (QuantizedConv2D) (None, 112, 112, 16) 448
conv_0/relu (QuantizedReLU) (None, 112, 112, 16) 32
conv_1 (QuantizedConv2D) (None, 112, 112, 32) 4640
conv_1/relu (QuantizedReLU) (None, 112, 112, 32) 64
conv_2 (QuantizedConv2D) (None, 56, 56, 64) 18496
conv_2/relu (QuantizedReLU) (None, 56, 56, 64) 128
conv_3 (QuantizedConv2D) (None, 56, 56, 64) 36928
conv_3/relu (QuantizedReLU) (None, 56, 56, 64) 128
dw_separable_4 (QuantizedDe (None, 28, 28, 64) 704
pthwiseConv2D)
pw_separable_4 (QuantizedCo (None, 28, 28, 128) 8320
nv2D)
pw_separable_4/relu (Quanti (None, 28, 28, 128) 256
zedReLU)
dw_separable_5 (QuantizedDe (None, 28, 28, 128) 1408
pthwiseConv2D)
pw_separable_5 (QuantizedCo (None, 28, 28, 128) 16512
nv2D)
pw_separable_5/relu (Quanti (None, 28, 28, 128) 256
zedReLU)
dw_separable_6 (QuantizedDe (None, 14, 14, 128) 1408
pthwiseConv2D)
pw_separable_6 (QuantizedCo (None, 14, 14, 256) 33024
nv2D)
pw_separable_6/relu (Quanti (None, 14, 14, 256) 512
zedReLU)
dw_separable_7 (QuantizedDe (None, 14, 14, 256) 2816
pthwiseConv2D)
pw_separable_7 (QuantizedCo (None, 14, 14, 256) 65792
nv2D)
pw_separable_7/relu (Quanti (None, 14, 14, 256) 512
zedReLU)
dw_separable_8 (QuantizedDe (None, 14, 14, 256) 2816
pthwiseConv2D)
pw_separable_8 (QuantizedCo (None, 14, 14, 256) 65792
nv2D)
pw_separable_8/relu (Quanti (None, 14, 14, 256) 512
zedReLU)
dw_separable_9 (QuantizedDe (None, 14, 14, 256) 2816
pthwiseConv2D)
pw_separable_9 (QuantizedCo (None, 14, 14, 256) 65792
nv2D)
pw_separable_9/relu (Quanti (None, 14, 14, 256) 512
zedReLU)
dw_separable_10 (QuantizedD (None, 14, 14, 256) 2816
epthwiseConv2D)
pw_separable_10 (QuantizedC (None, 14, 14, 256) 65792
onv2D)
pw_separable_10/relu (Quant (None, 14, 14, 256) 512
izedReLU)
dw_separable_11 (QuantizedD (None, 14, 14, 256) 2816
epthwiseConv2D)
pw_separable_11 (QuantizedC (None, 14, 14, 256) 65792
onv2D)
pw_separable_11/relu (Quant (None, 14, 14, 256) 512
izedReLU)
dw_separable_12 (QuantizedD (None, 7, 7, 256) 2816
epthwiseConv2D)
pw_separable_12 (QuantizedC (None, 7, 7, 512) 131584
onv2D)
pw_separable_12/relu (Quant (None, 7, 7, 512) 1024
izedReLU)
dw_separable_13 (QuantizedD (None, 7, 7, 512) 5632
epthwiseConv2D)
pw_separable_13 (QuantizedC (None, 7, 7, 512) 262656
onv2D)
pw_separable_13/relu (Quant (None, 7, 7, 512) 1024
izedReLU)
dw_1conv (QuantizedDepthwis (None, 7, 7, 512) 5632
eConv2D)
pw_1conv (QuantizedConv2D) (None, 7, 7, 1024) 525312
pw_1conv/relu (QuantizedReL (None, 7, 7, 1024) 2048
U)
dw_2conv (QuantizedDepthwis (None, 7, 7, 1024) 11264
eConv2D)
pw_2conv (QuantizedConv2D) (None, 7, 7, 1024) 1049600
pw_2conv/relu (QuantizedReL (None, 7, 7, 1024) 2048
U)
dw_3conv (QuantizedDepthwis (None, 7, 7, 1024) 11264
eConv2D)
pw_3conv (QuantizedConv2D) (None, 7, 7, 1024) 1049600
pw_3conv/relu (QuantizedReL (None, 7, 7, 1024) 2048
U)
dw_detection_layer (Quantiz (None, 7, 7, 1024) 11264
edDepthwiseConv2D)
pw_detection_layer (Quantiz (None, 7, 7, 35) 35875
edConv2D)
dequantizer (Dequantizer) (None, 7, 7, 35) 0
=================================================================
Total params: 3,579,555
Trainable params: 3,555,523
Non-trainable params: 24,032
_________________________________________________________________
# Define the final reshape and build the model
output = Reshape((grid_size[1], grid_size[0], num_anchors, 4 + 1 + classes),
name="YOLO_output")(model_keras.output)
model_keras = Model(model_keras.input, output)
# Create the mAP evaluator object
num_images = 100
map_evaluator = MapEvaluation(model_keras, val_data[:num_images], labels,
anchors)
# Compute the scores for all validation images
start = timer()
mAP, average_precisions = map_evaluator.evaluate_map()
end = timer()
for label, average_precision in average_precisions.items():
print(labels[label], '{:.4f}'.format(average_precision))
print('mAP: {:.4f}'.format(mAP))
print(f'Keras inference on {num_images} images took {end-start:.2f} s.\n')
car 0.4726
person 0.4384
mAP: 0.4555
Keras inference on 100 images took 13.36 s.
6. Conversion to Akida
6.1 Convert to Akida model
The last YOLO_output layer that was added for splitting channels into values for each box must be removed before Akida conversion.
# Rebuild a model without the last layer
compatible_model = Model(model_keras.input, model_keras.layers[-2].output)
When converting to an Akida model, we just need to pass the Keras model to cnn2snn.convert.
from cnn2snn import convert
model_akida = convert(compatible_model)
model_akida.summary()
Model Summary
________________________________________________
Input shape Output shape Sequences Layers
================================================
[224, 224, 3] [7, 7, 35] 1 33
________________________________________________
__________________________________________________________________________
Layer (type) Output shape Kernel shape
==================== SW/conv_0-dequantizer (Software) ====================
conv_0 (InputConv2D) [112, 112, 16] (3, 3, 3, 16)
__________________________________________________________________________
conv_1 (Conv2D) [112, 112, 32] (3, 3, 16, 32)
__________________________________________________________________________
conv_2 (Conv2D) [56, 56, 64] (3, 3, 32, 64)
__________________________________________________________________________
conv_3 (Conv2D) [56, 56, 64] (3, 3, 64, 64)
__________________________________________________________________________
dw_separable_4 (DepthwiseConv2D) [28, 28, 64] (3, 3, 64, 1)
__________________________________________________________________________
pw_separable_4 (Conv2D) [28, 28, 128] (1, 1, 64, 128)
__________________________________________________________________________
dw_separable_5 (DepthwiseConv2D) [28, 28, 128] (3, 3, 128, 1)
__________________________________________________________________________
pw_separable_5 (Conv2D) [28, 28, 128] (1, 1, 128, 128)
__________________________________________________________________________
dw_separable_6 (DepthwiseConv2D) [14, 14, 128] (3, 3, 128, 1)
__________________________________________________________________________
pw_separable_6 (Conv2D) [14, 14, 256] (1, 1, 128, 256)
__________________________________________________________________________
dw_separable_7 (DepthwiseConv2D) [14, 14, 256] (3, 3, 256, 1)
__________________________________________________________________________
pw_separable_7 (Conv2D) [14, 14, 256] (1, 1, 256, 256)
__________________________________________________________________________
dw_separable_8 (DepthwiseConv2D) [14, 14, 256] (3, 3, 256, 1)
__________________________________________________________________________
pw_separable_8 (Conv2D) [14, 14, 256] (1, 1, 256, 256)
__________________________________________________________________________
dw_separable_9 (DepthwiseConv2D) [14, 14, 256] (3, 3, 256, 1)
__________________________________________________________________________
pw_separable_9 (Conv2D) [14, 14, 256] (1, 1, 256, 256)
__________________________________________________________________________
dw_separable_10 (DepthwiseConv2D) [14, 14, 256] (3, 3, 256, 1)
__________________________________________________________________________
pw_separable_10 (Conv2D) [14, 14, 256] (1, 1, 256, 256)
__________________________________________________________________________
dw_separable_11 (DepthwiseConv2D) [14, 14, 256] (3, 3, 256, 1)
__________________________________________________________________________
pw_separable_11 (Conv2D) [14, 14, 256] (1, 1, 256, 256)
__________________________________________________________________________
dw_separable_12 (DepthwiseConv2D) [7, 7, 256] (3, 3, 256, 1)
__________________________________________________________________________
pw_separable_12 (Conv2D) [7, 7, 512] (1, 1, 256, 512)
__________________________________________________________________________
dw_separable_13 (DepthwiseConv2D) [7, 7, 512] (3, 3, 512, 1)
__________________________________________________________________________
pw_separable_13 (Conv2D) [7, 7, 512] (1, 1, 512, 512)
__________________________________________________________________________
dw_1conv (DepthwiseConv2D) [7, 7, 512] (3, 3, 512, 1)
__________________________________________________________________________
pw_1conv (Conv2D) [7, 7, 1024] (1, 1, 512, 1024)
__________________________________________________________________________
dw_2conv (DepthwiseConv2D) [7, 7, 1024] (3, 3, 1024, 1)
__________________________________________________________________________
pw_2conv (Conv2D) [7, 7, 1024] (1, 1, 1024, 1024)
__________________________________________________________________________
dw_3conv (DepthwiseConv2D) [7, 7, 1024] (3, 3, 1024, 1)
__________________________________________________________________________
pw_3conv (Conv2D) [7, 7, 1024] (1, 1, 1024, 1024)
__________________________________________________________________________
dw_detection_layer (DepthwiseConv2D) [7, 7, 1024] (3, 3, 1024, 1)
__________________________________________________________________________
pw_detection_layer (Conv2D) [7, 7, 35] (1, 1, 1024, 35)
__________________________________________________________________________
dequantizer (Dequantizer) [7, 7, 35] N/A
__________________________________________________________________________
6.1 Check performance
Akida model accuracy is tested on the first n images of the validation set.
# Create the mAP evaluator object
map_evaluator_ak = MapEvaluation(model_akida,
val_data[:num_images],
labels,
anchors,
is_keras_model=False)
# Compute the scores for all validation images
start = timer()
mAP_ak, average_precisions_ak = map_evaluator_ak.evaluate_map()
end = timer()
for label, average_precision in average_precisions_ak.items():
print(labels[label], '{:.4f}'.format(average_precision))
print('mAP: {:.4f}'.format(mAP_ak))
print(f'Akida inference on {num_images} images took {end-start:.2f} s.\n')
car 0.4997
person 0.4164
mAP: 0.4580
Akida inference on 100 images took 12.84 s.
6.2 Show predictions for a random image
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.patches as patches
from akida_models.detection.processing import load_image, preprocess_image, decode_output
# Take a random test image
i = np.random.randint(len(val_data))
input_shape = model_akida.layers[0].input_dims
# Load the image
raw_image = load_image(val_data[i]['image_path'])
# Keep the original image size for later bounding boxes rescaling
raw_height, raw_width, _ = raw_image.shape
# Pre-process the image
image = preprocess_image(raw_image, input_shape)
input_image = image[np.newaxis, :].astype(np.uint8)
# Call evaluate on the image
pots = model_akida.predict(input_image)[0]
# Reshape the potentials to prepare for decoding
h, w, c = pots.shape
pots = pots.reshape((h, w, len(anchors), 4 + 1 + len(labels)))
# Decode potentials into bounding boxes
raw_boxes = decode_output(pots, anchors, len(labels))
# Rescale boxes to the original image size
pred_boxes = np.array([[
box.x1 * raw_width, box.y1 * raw_height, box.x2 * raw_width,
box.y2 * raw_height,
box.get_label(),
box.get_score()
] for box in raw_boxes])
fig = plt.figure(num='VOC2012 car and person detection by Akida')
ax = fig.subplots(1)
img_plot = ax.imshow(np.zeros(raw_image.shape, dtype=np.uint8))
img_plot.set_data(raw_image)
for box in pred_boxes:
rect = patches.Rectangle((box[0], box[1]),
box[2] - box[0],
box[3] - box[1],
linewidth=1,
edgecolor='r',
facecolor='none')
ax.add_patch(rect)
class_score = ax.text(box[0],
box[1] - 5,
f"{labels[int(box[4])]} - {box[5]:.2f}",
color='red')
plt.axis('off')
plt.show()
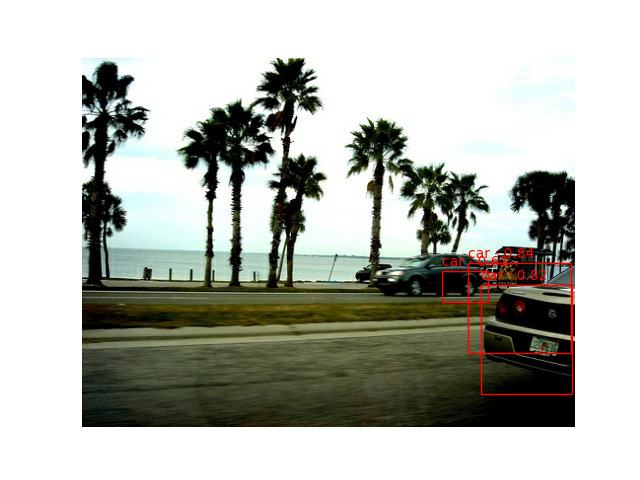
Total running time of the script: (1 minutes 25.192 seconds)